#1 - Basic Fundamentals - DSA Series With C++ | Akash Vishwakarma
1. Syntax and Control Structures
Basic Syntax
Every C++ program starts with a main function:
int main() { // Your code here return 0; }
Variables and Data Types
- Integer types: int, short, long, long long
- Floating-point types: float, double, long double
- Character types: char
- Boolean type: bool
- Void type
Control Structures
If-else Statements
if (condition) { // code block } else if (another_condition) { // code block } else { // code block }
Loops
C++ supports three main types of loops:
// For loop for (int i = 0; i < n; i++) { // code block } // While loop while (condition) { // code block } // Do-while loop do { // code block } while (condition);
2. Functions and Recursion
Functions
Functions are blocks of reusable code that perform specific tasks:
return_type function_name(parameters) { // function body return value; }
Function Overloading
C++ allows multiple functions with the same name but different parameters:
int add(int a, int b) { return a + b; } double add(double a, double b) { return a + b; }
Recursion
Recursion is when a function calls itself. Here's a classic example of calculating factorial:
int factorial(int n) { if (n <= 1) return 1; return n * factorial(n - 1); }
3. Pointers and Dynamic Memory Allocation
Pointers
Pointers store memory addresses of variables:
int x = 10; int* ptr = &x; // & operator gets address of x cout << *ptr; // * operator dereferences pointer
Dynamic Memory Allocation
Use new and delete operators for dynamic memory management:
// Single element int* p = new int; *p = 10; delete p; // Array int* arr = new int[5]; // use array delete[] arr;
4. Object-Oriented Programming (OOP)
Classes and Objects
class Person { private: string name; int age; public: Person(string n, int a) : name(n), age(a) {} void displayInfo() { cout << "Name: " << name << ", Age: " << age << endl; } };
Inheritance
class Student : public Person { private: string studentId; public: Student(string n, int a, string id) : Person(n, a), studentId(id) {} };
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common base class:
class Shape { public: virtual double area() = 0; // Pure virtual function }; class Circle : public Shape { private: double radius; public: double area() override { return 3.14159 * radius * radius; } };
5. Exception Handling and File I/O
Exception Handling
try { // code that might throw an exception if (someErrorCondition) { throw runtime_error("Error message"); } } catch (const exception& e) { cout << "Error: " << e.what() << endl; }
File I/O
#include // Writing to a file ofstream outFile("example.txt"); outFile << "Hello, World!" << endl; outFile.close(); // Reading from a file ifstream inFile("example.txt"); string line; while (getline(inFile, line)) { cout << line << endl; } inFile.close();
Common Interview Questions
Q1: What is the difference between references and pointers in C++?
Answer: References are aliases for existing variables and must be initialized when declared. They cannot be null and cannot be reassigned. Pointers can be null, can be reassigned, and need to be dereferenced using the * operator.
Q2: Explain the concept of virtual functions.
Answer: Virtual functions enable runtime polymorphism in C++. They allow a base class pointer to call the correct derived class function based on the actual object type at runtime. They are declared using the 'virtual' keyword in the base class and can be overridden in derived classes.
Q3: What is the difference between stack and heap memory?
Answer: Stack memory is automatically managed and stores local variables and function calls. It's faster but limited in size. Heap memory is manually managed using new/delete operators, is larger but slower, and can lead to memory leaks if not properly managed.
Q4: Explain the concept of function overloading.
Answer: Function overloading allows multiple functions with the same name but different parameters (number, types, or both) to coexist. The compiler determines which function to call based on the arguments provided at compile time.
Q5: What are the access specifiers in C++ and their significance?
Answer: C++ has three access specifiers: public (accessible from anywhere), private (accessible only within the class), and protected (accessible within the class and its derived classes). They help in implementing encapsulation and data hiding.
Keywords
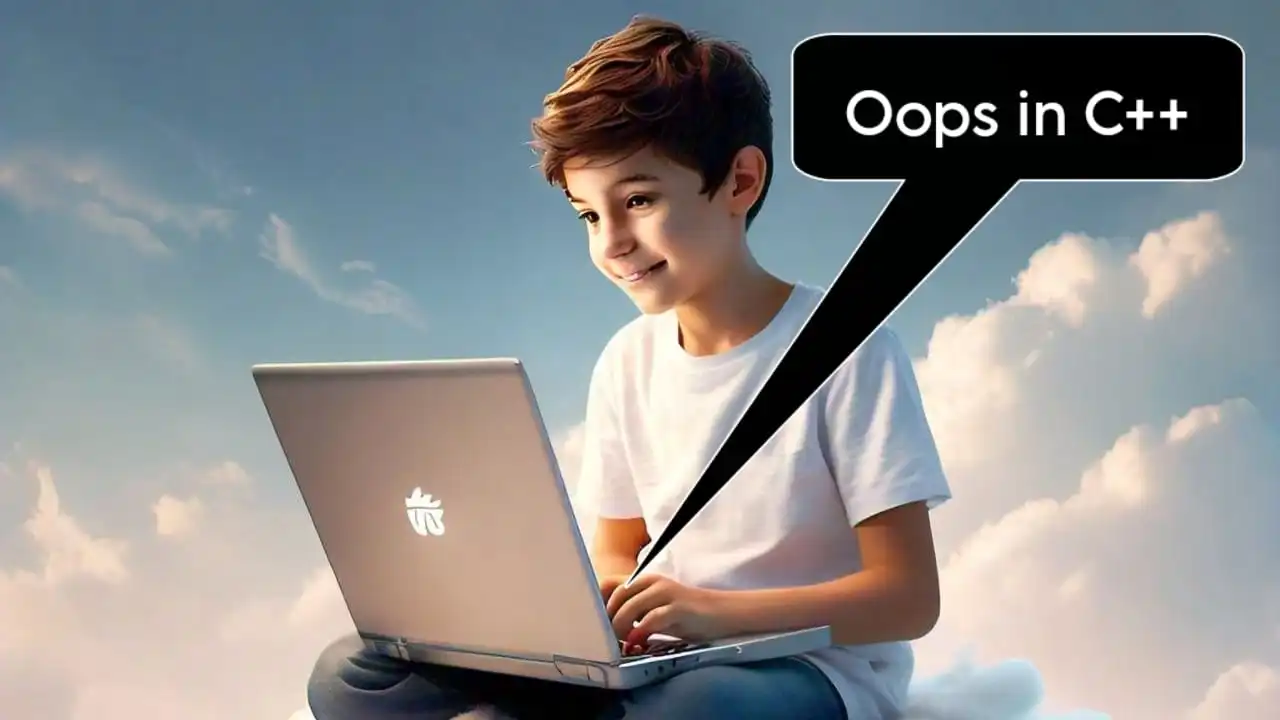
OOPs (Object Oriented Programming System) | Concepts & Interview Question with Examples
The major purpose of C++ programming is to introduce the concept of object orientation to the C programming language. Object Oriented Programming is a paradigm that provides many concepts such as inhe
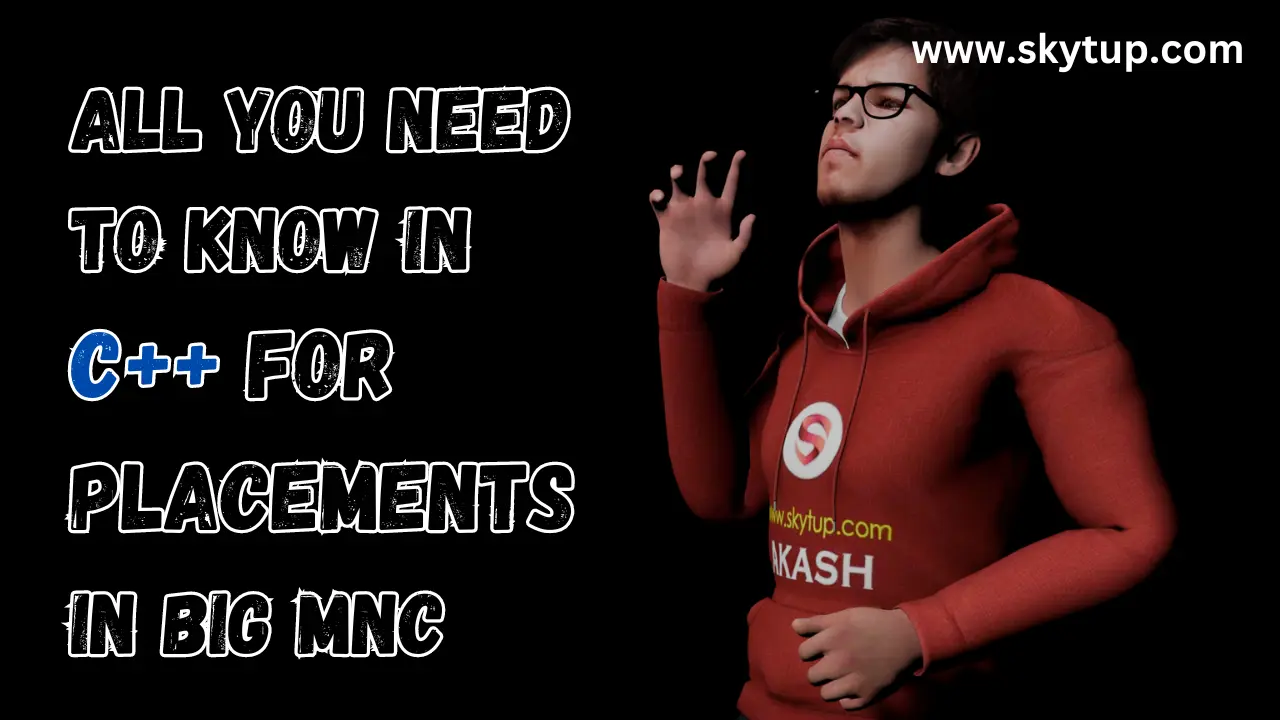
All you need to know in C++ for placement in big MNC | Requirements and eligibility criteria
Boost your chances of landing a job at top MNCs with expertise in C++. Learn the required skills, data structures, algorithms, and eligibility criteria to excel in placement tests. Stay ahead with our
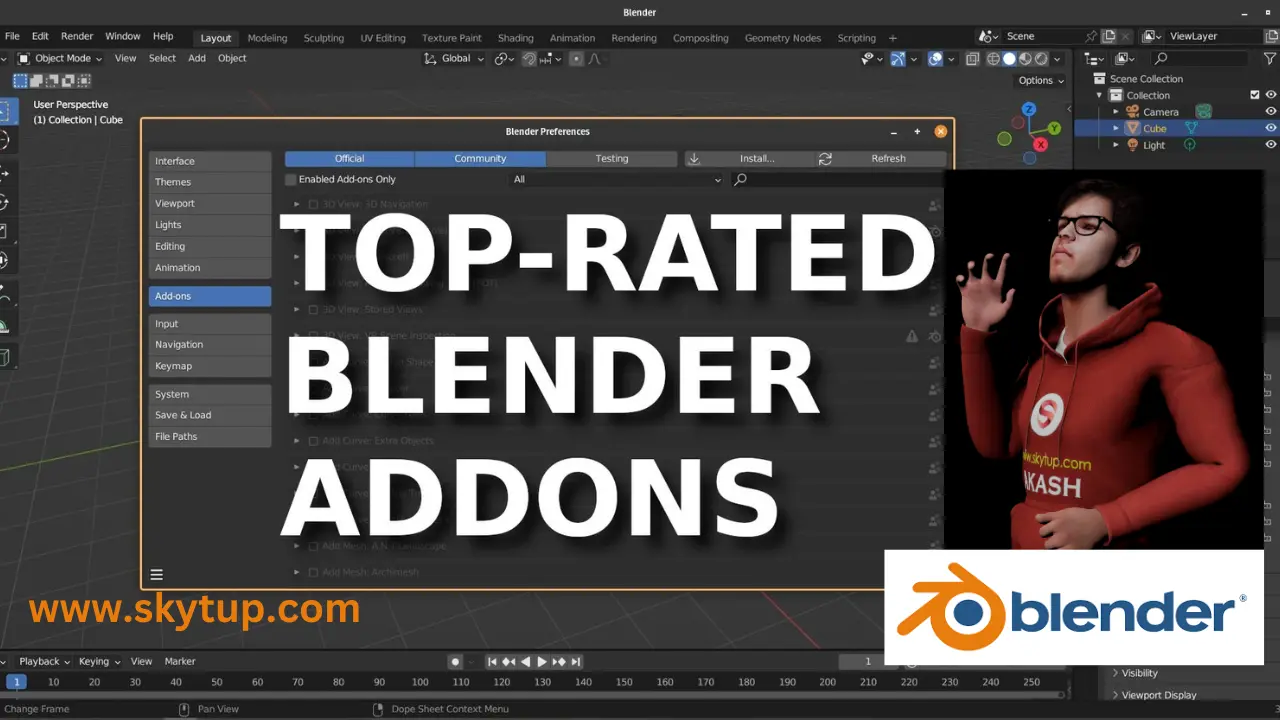
Top 15 Blender Add-ons for Becoming a Pro in the 3D World π₯
"Take your 3D skills to the next level with these top 10 Blender add-ons. From modeling and texturing to animation and rendering, discover the best tools to streamline your workflow and create s
Performance Comparison of Programming Languages: Counting from 1 to 1 Billion
This article compares the performance of various programming languages, including C, C++, Java, Python, Go, C#, and JavaScript, by measuring the time each takes to count from 1 to 1 billion. Detailed
LeetCode Problem π₯ 1945 - Sum of Digits of String After Convert
This type of problem is common in competitive programming and technical interviews, as it tests your understanding of basic string manipulation, numeric transformations, and iterative processes. In th

Building a Distance-Measuring LED Indicator Using Arduino and Ultrasonic Sensor π₯
Learn to create a distance-measuring LED indicator using an Arduino and an HC-SR04 ultrasonic sensor. This project guides you through setting up the sensor to control LEDs based on object proximity, o
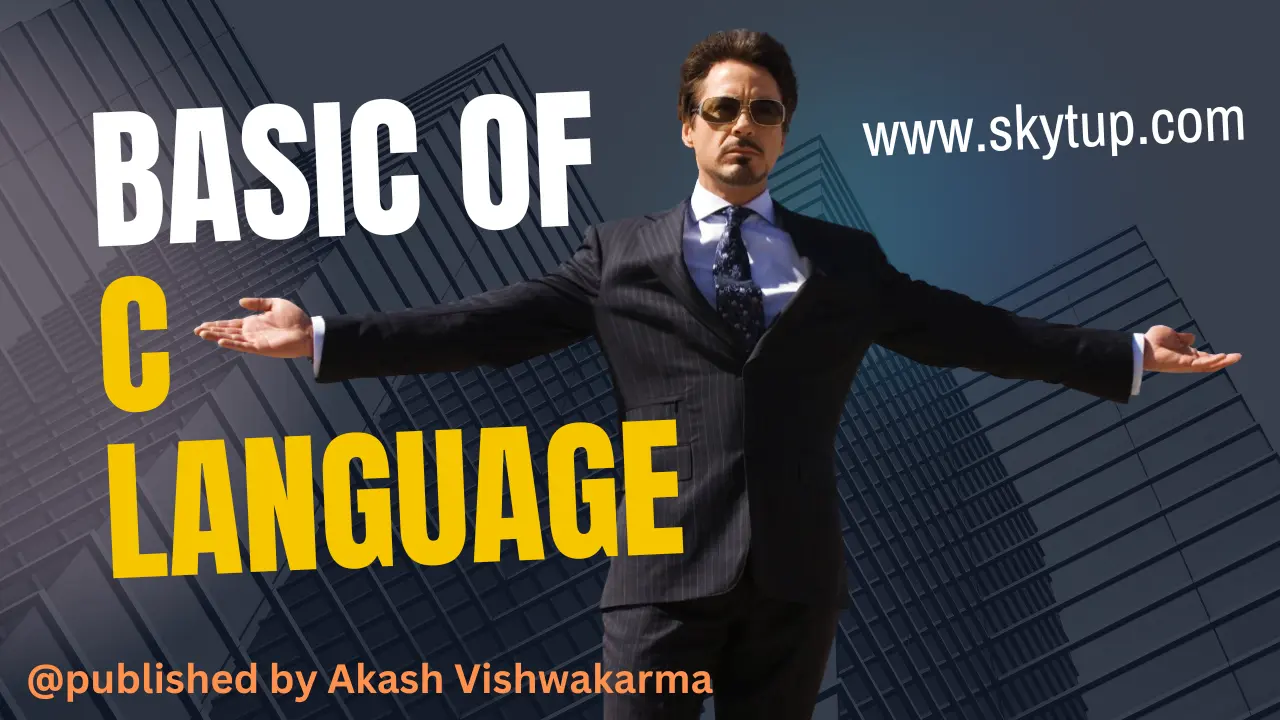
Basics of C Language for beginners | Syntax and Common Interview questionπ₯
C is a procedural programming language with a static system that has the functionality of structured programming, recursion, and lexical variable scoping. C was created with constructs that transfer w
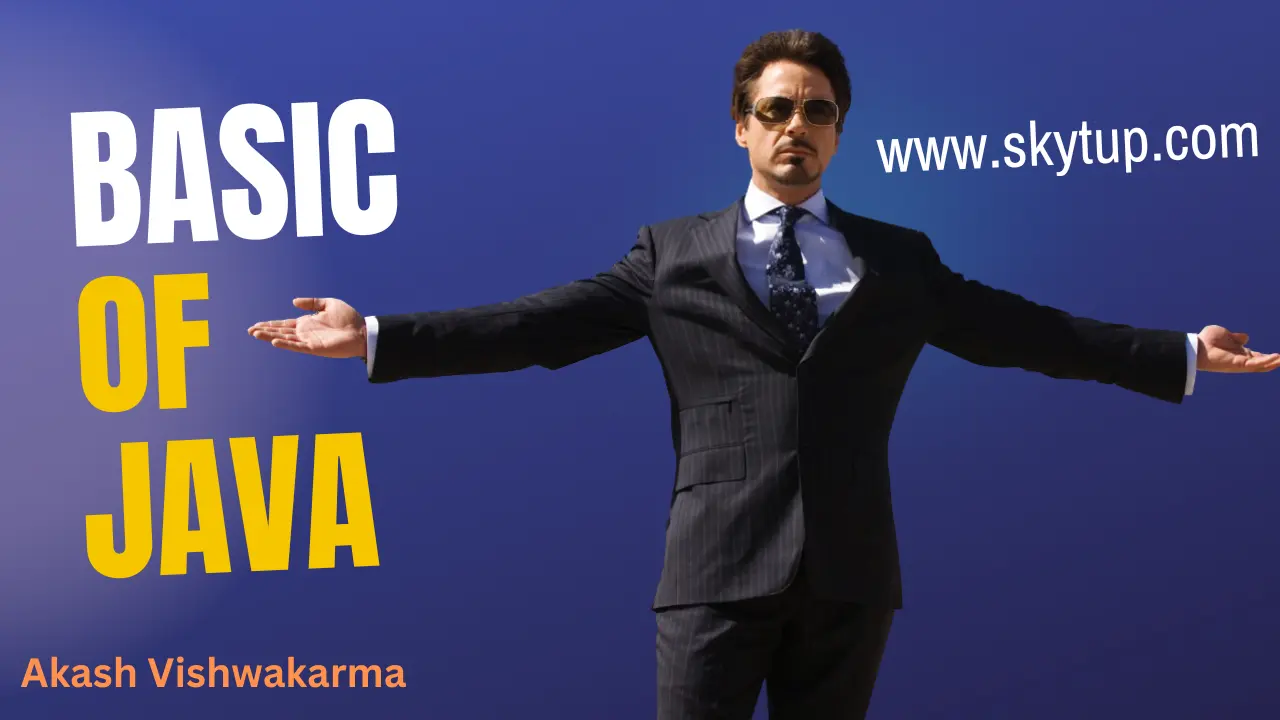
Basics of Java Programming and common Interview Questions π₯
Java Platform is a collection of programs. It helps to develop and run a program written in the Java programming language. Java Platform includes an execution engine, a compiler and set of libraries.
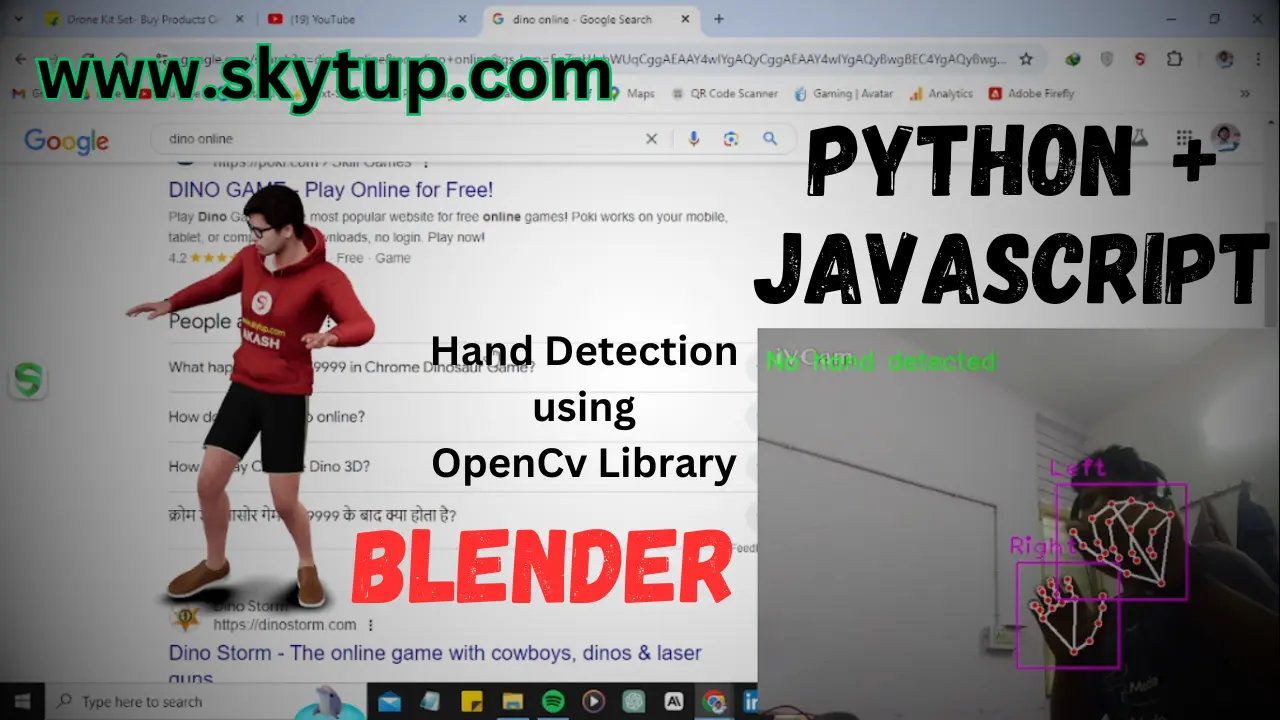
Hand Gesture Control Using OpenCV and Cvzone | Python Project by Akash Vishwakarma
Hey I am Akash Vishwakarma in this article I am gonna show you hand detection system using python with opencv library. This is an open source library which you can use to detect multiple hand gestures
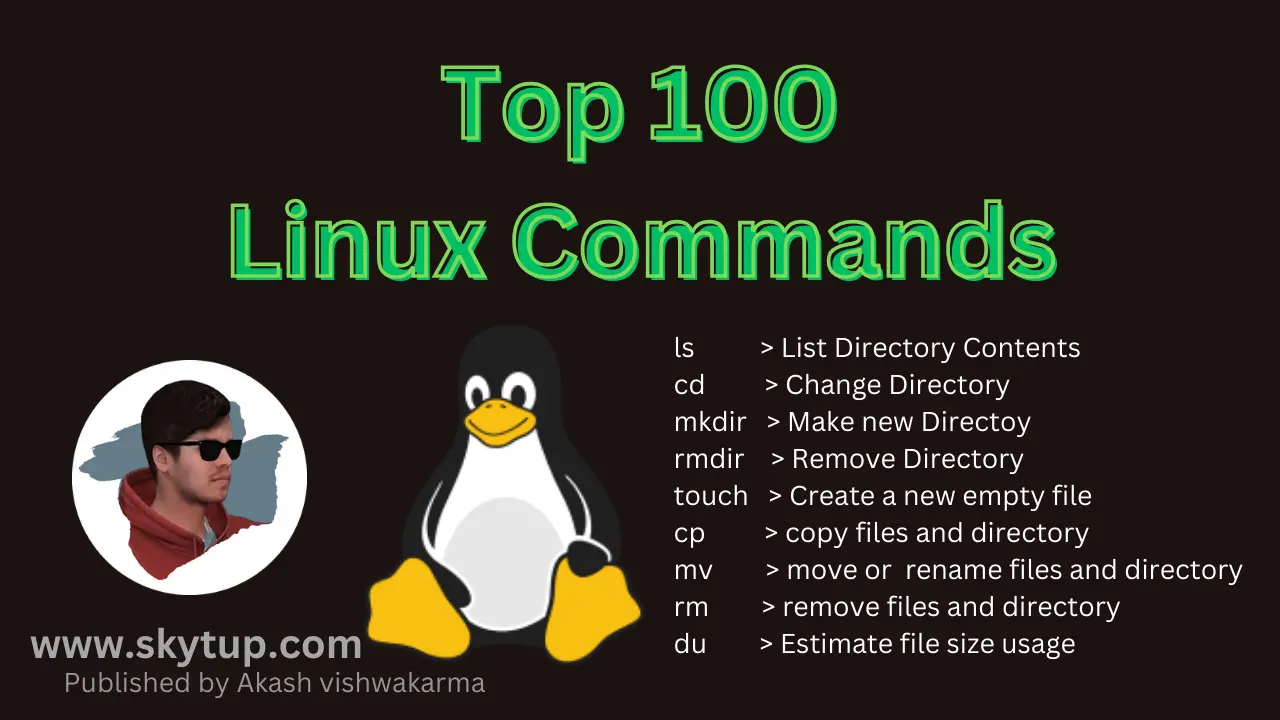
Top 100 Linux Commands you Must know in 2024 | Akash
Hi Everyone, My name is Akash in this article I you will learn top 100 linux commands. By mastering these essential Linux commands, you'll become more efficient and confident in your ability to w
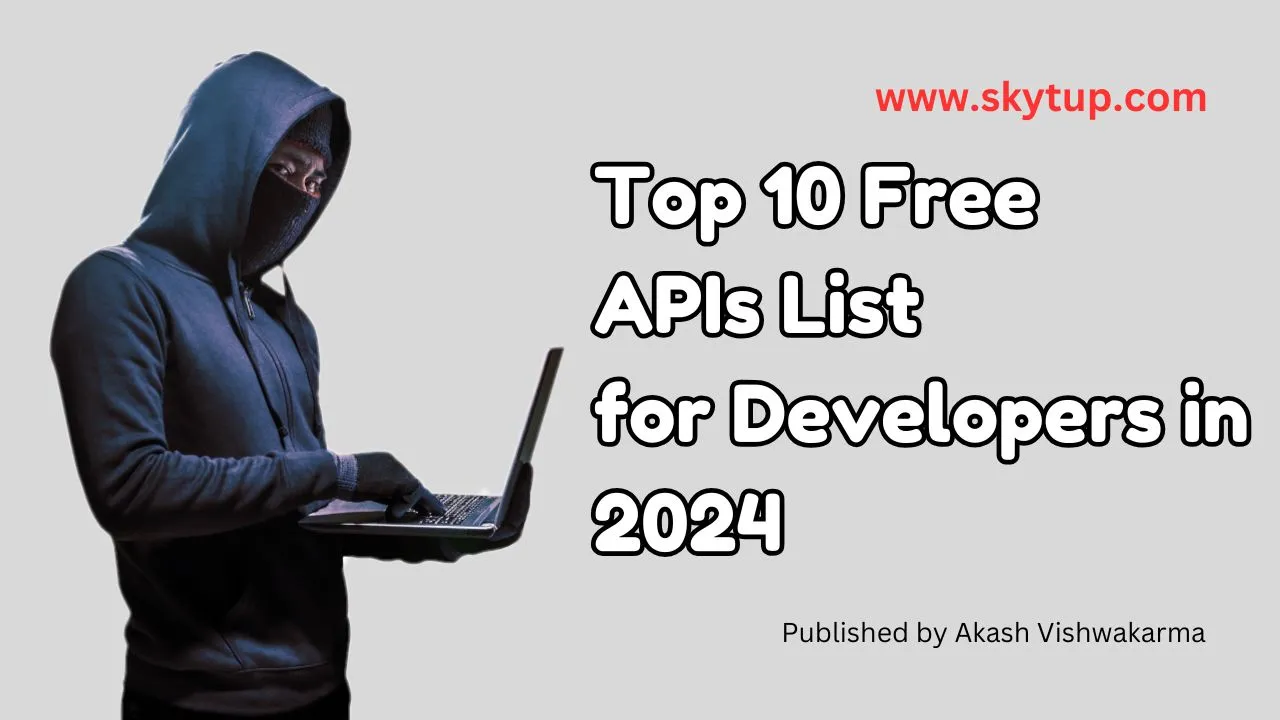
Top 10 Free APIs with Documentation for Developers in 2024 π₯
Hi Everyone this is Akash Vishwakarma. In this Article we have Top 10 Api List by using these Api Endpoints You can make Amazing Projects and showcase them on your portfolio. You can show these to rec
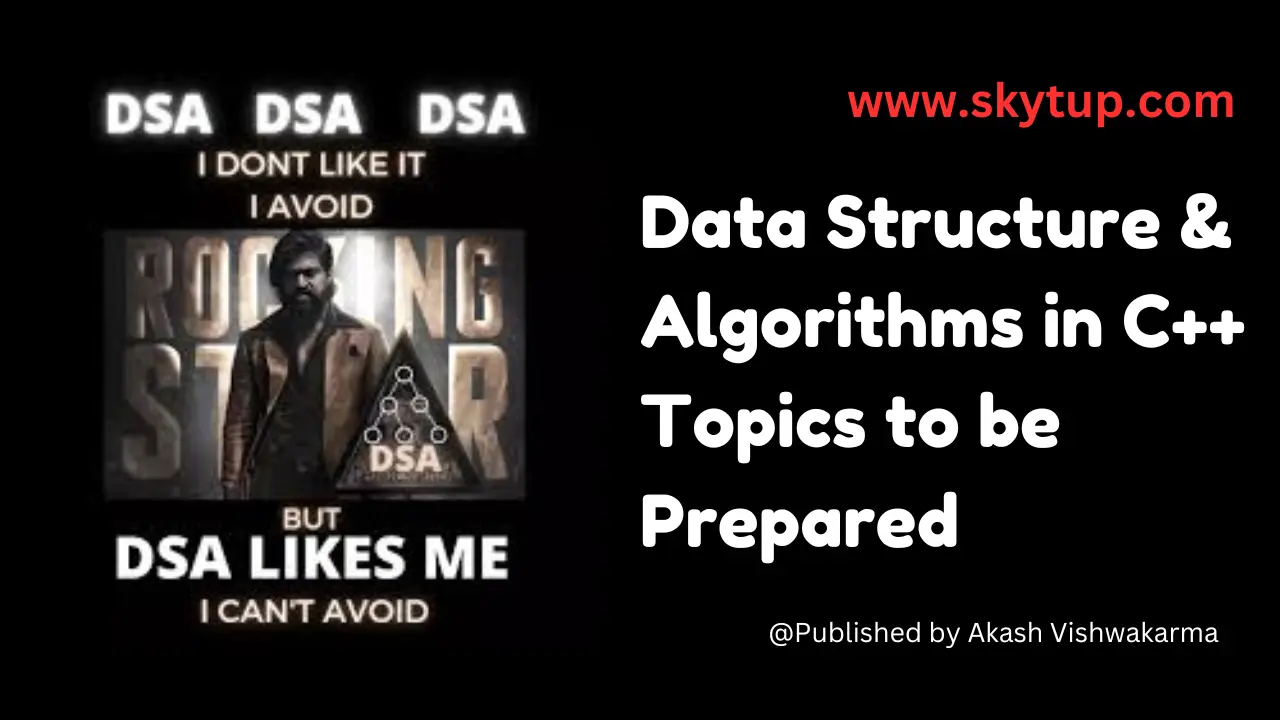
Data Structures and Algorithms in C++ | Topics to be prepared for Interviews
Hi everyone, I'm Akash Vishwakarma. As someone who passionate about programming, I've often found myself wondering what it takes to crack those tough technical interviews at top tech
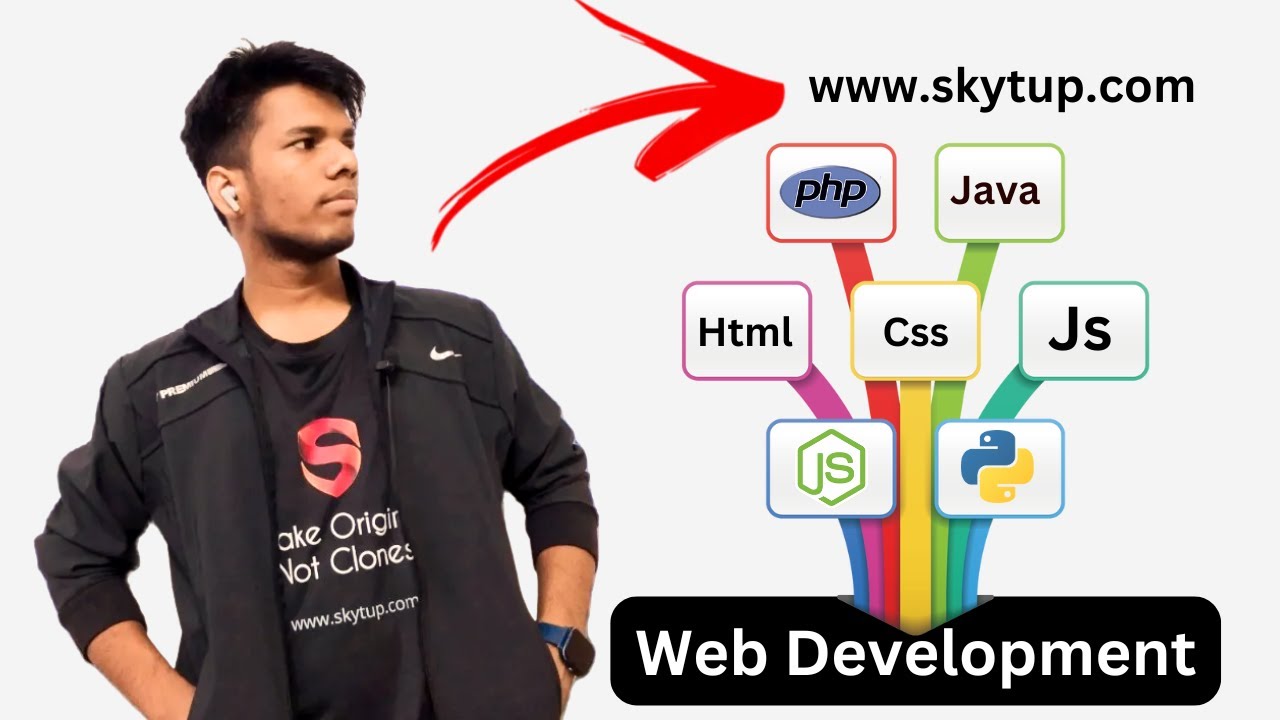
Become a Full Stack Web Developer | A Quick Roadmap for Beginners in 2025 | Developer Akash
Hi Everyone,its me Akash, In this Article I&amp;#039;ve Shared a Roadmap to become a Full Stack Web Developer. Follow guildines given in this article and you will must know something new and i

Advanced Guide to C++ STL for Competitive Programming
The C++ STL is built on three fundamental components: containers, algorithms, and iterators. Understanding how these components interact and their underlying implementations is crucial for optimal usa
#1 - Basic Fundamentals - DSA Series With C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is 1st Article of DSA Series on Skytup. In this Article We will learn about C++ Fundamentals. This article is written with the help of Ai Tools and some of my own exp
#2 - Arrays and Strings - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #2 of Our DSA Series on Skytup. In this Article We will learn about C++ Fundamentals. This article is written with the help of Ai Tools and some of my o
#3 - Linked Lists - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #3 of Our DSA Series on Skytup. In this Article We will learn about Linked Lists. This article is written with the help of Ai Tools and some of my own e
#4 - Stacks and Queues - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #4 of Our DSA Series on Skytup. In this Article We will learn about C++ Stack and Queues. This article is written with the help of Ai Tools and some of
#5 - Trees - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #5 of Our DSA Series on Skytup. In this Article We will learn about C++ Trees. This article is written with the help of Ai Tools and some of my own expe