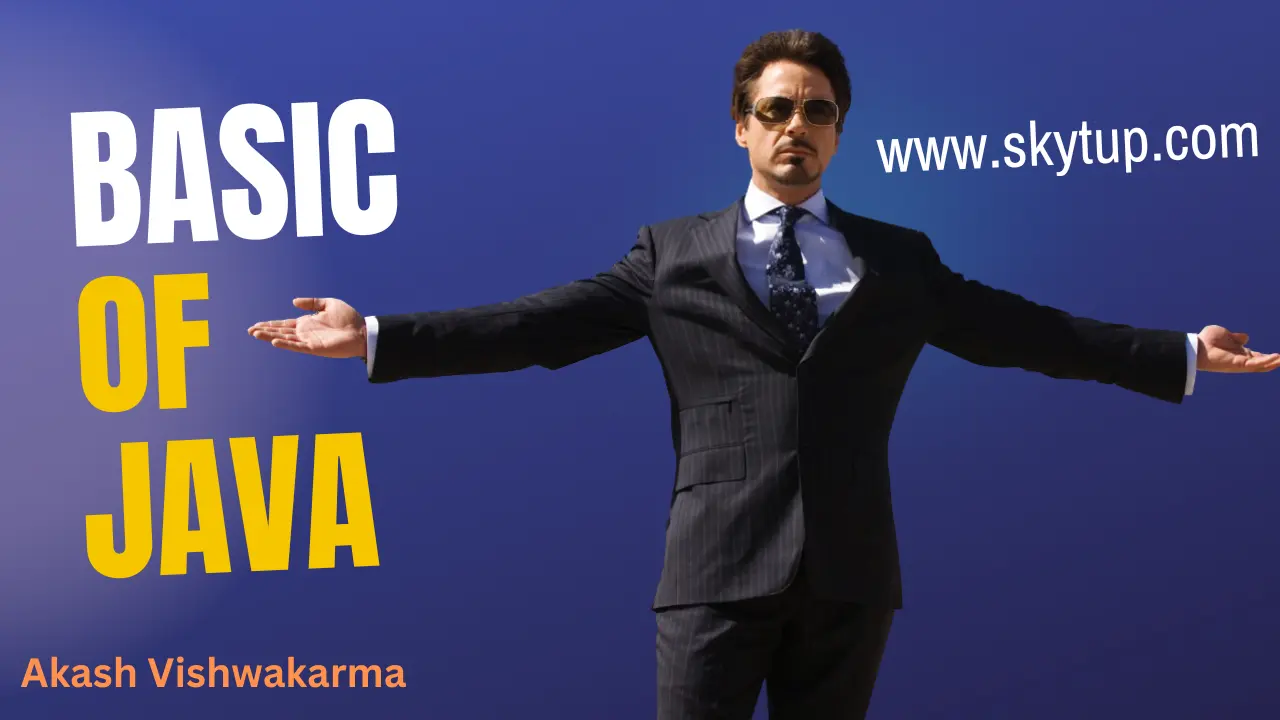
Basics of Java Programming and common Interview Questions π₯
Table of Contents
- Introduction to Java
- History and Evolution
- Key Features of Java
- Setting Up the Development Environment
- Structure of a Java Program
- Data Types and Variables
- Operators
- Control Structures
- Object-Oriented Programming in Java
- Arrays and Collections
- Exception Handling
- Input/Output Operations
- Multithreading
- Generics
- Lambda Expressions and Functional Interfaces
- Streams API
- Java Module System
- Best Practices and Coding Standards
- Common Interview Questions
- Conclusion
1. Introduction to Java
Java is a versatile, object-oriented programming language designed to be platform-independent. It follows the principle of "Write Once, Run Anywhere" (WORA), allowing Java applications to run on any device with a Java Virtual Machine (JVM) installed.
Key characteristics of Java include:
- Object-oriented
- Platform-independent
- Secure
- Robust
- Multithreaded
- High performance
- Distributed
- Dynamic
2. History and Evolution
Java has a rich history spanning several decades:
- 1991: James Gosling and his team at Sun Microsystems begin work on Java
- 1995: Java 1.0 is officially released
- 1998: Java 2 (J2SE 1.2) introduces significant improvements
- 2006: Java becomes open-source
- 2010: Oracle acquires Sun Microsystems
- 2014: Java 8 released with lambda expressions and the Stream API
- 2017: Java 9 introduces the module system
- 2018 onwards: Six-month release cycle begins
3. Key Features of Java
- Platform Independence: Java's "Write Once, Run Anywhere" philosophy
- Object-Oriented: Everything in Java is an object, facilitating modular and flexible code
- Robust: Strong type checking, exception handling, and automatic memory management
- Secure: Security manager for defining access rules
- Multithreaded: Built-in support for concurrent programming
- High Performance: Just-In-Time compiler for improved speed
- Distributed: Extensive networking capabilities
- Dynamic: Runtime class loading and reflection capabilities
4. Setting Up the Development Environment
To start programming in Java, you need to set up a development environment:
- Install Java Development Kit (JDK):
- Download and install the latest JDK from Oracle or OpenJDK
- Set up JAVA_HOME environment variable
- Add Java's bin directory to the system PATH
- Choose an Integrated Development Environment (IDE):
- Eclipse
- IntelliJ IDEA
- NetBeans
- Visual Studio Code with Java extensions
- Verify the installation:
java -version javac -version
5. Structure of a Java Program
A basic Java program structure looks like this:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Let's break down the structure:
public class HelloWorld
: Class declarationpublic static void main(String[] args)
: Main method, the entry point of the programSystem.out.println()
: Method to print output to the console
6. Data Types and Variables
Java is a statically-typed language and supports two categories of data types:
Primitive Data Types
- byte: 8-bit signed two's complement integer
- short: 16-bit signed two's complement integer
- int: 32-bit signed two's complement integer
- long: 64-bit signed two's complement integer
- float: 32-bit IEEE 754 floating point
- double: 64-bit IEEE 754 floating point
- boolean: true or false
- char: 16-bit Unicode character
Reference Data Types
- Classes
- Interfaces
- Arrays
Variable Declaration and Initialization
int age = 30;
double salary = 50000.50;
String name = "John Doe";
7. Operators
Java provides various types of operators:
- Arithmetic operators:
+
,-
,*
,/
,%
- Relational operators:
==
,!=
,<
,>
,<=
,>=
- Logical operators:
&&
,||
,!
- Bitwise operators:
&
,|
,^
,~
,<<
,>>
,>>>
- Assignment operators:
=
,+=
,-=
,*=
,/=
,%=
, etc. - Increment/Decrement operators:
++
,--
- Conditional operator:
?:
- instanceof operator
8. Control Structures
Java provides several control structures for decision-making and looping:
Conditional Statements
// If statement
if (condition) {
// code
} else if (anotherCondition) {
// code
} else {
// code
}
// Switch statement
switch (expression) {
case value1:
// code
break;
case value2:
// code
break;
default:
// code
}
Loops
// For loop
for (initialization; condition; update) {
// code
}
// Enhanced for loop (for-each)
for (Type item : collection) {
// code
}
// While loop
while (condition) {
// code
}
// Do-while loop
do {
// code
} while (condition);
9. Object-Oriented Programming in Java
Java is fundamentally object-oriented and supports all the main concepts of OOP:
Classes and Objects
public class Car {
private String brand;
private String model;
public Car(String brand, String model) {
this.brand = brand;
this.model = model;
}
public void start() {
System.out.println("The " + brand + " " + model + " is starting.");
}
}
// Creating an object
Car myCar = new Car("Toyota", "Corolla");
myCar.start();
Inheritance
public class ElectricCar extends Car {
private int batteryCapacity;
public ElectricCar(String brand, String model, int batteryCapacity) {
super(brand, model);
this.batteryCapacity = batteryCapacity;
}
@Override
public void start() {
super.start();
System.out.println("Electric motor activated.");
}
}
Polymorphism
Car car1 = new Car("Honda", "Civic");
Car car2 = new ElectricCar("Tesla", "Model 3", 75);
car1.start(); // Calls Car's start method
car2.start(); // Calls ElectricCar's start method
Encapsulation
Achieved through access modifiers (public, private, protected) and getter/setter methods.
Abstraction
public abstract class Vehicle {
public abstract void move();
}
public class Bicycle extends Vehicle {
@Override
public void move() {
System.out.println("Pedaling the bicycle.");
}
}
Interfaces
public interface Chargeable {
void charge();
}
public class ElectricCar extends Car implements Chargeable {
@Override
public void charge() {
System.out.println("Charging the electric car.");
}
}
10. Arrays and Collections
Arrays
int[] numbers = new int[5];
int[] primes = {2, 3, 5, 7, 11};
// Multidimensional array
int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Collections Framework
Java provides a rich set of collection classes:
- List: ArrayList, LinkedList
- Set: HashSet, TreeSet
- Queue: PriorityQueue
- Map: HashMap, TreeMap
import java.util.*;
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
Set<Integer> uniqueNumbers = new HashSet<>();
uniqueNumbers.add(1);
uniqueNumbers.add(2);
Map<String, Integer> ages = new HashMap<>();
ages.put("Alice", 30);
ages.put("Bob", 25);
11. Exception Handling
Java uses a try-catch-finally block structure for exception handling:
try {
// Code that may throw an exception
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero: " + e.getMessage());
} finally {
System.out.println("This block always executes");
}
// Custom exceptions
class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
// Throwing an exception
if (someCondition) {
throw new CustomException("This is a custom exception");
}
12. Input/Output Operations
Java provides various classes for I/O operations:
File I/O
import java.io.*;
// Writing to a file
try (FileWriter writer = new FileWriter("output.txt")) {
writer.write("Hello, World!");
} catch (IOException e) {
e.printStackTrace();
}
// Reading from a file
try (BufferedReader reader = new BufferedReader(new FileReader("input.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
Console I/O
import java.util.Scanner;
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
System.out.println("Hello, " + name + "!");
scanner.close();
13. Multithreading
Java supports multithreading for concurrent programming:
// Extending Thread class
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running");
}
}
MyThread thread = new MyThread();
thread.start();
// Implementing Runnable interface
class MyRunnable implements Runnable {
public void run() {
System.out.println("Runnable is running ");
}
}
Thread thread = new Thread(new MyRunnable());
thread.start();
// Using ExecutorService
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
ExecutorService executor = Executors.newFixedThreadPool(5);
executor.submit(() -> {
System.out.println("Task executed by ExecutorService");
});
executor.shutdown();
14. Generics
Generics enable you to write reusable, type-safe code:
// Generic class
public class Box<T> {
private T content;
public void set(T content) {
this.content = content;
}
public T get() {
return content;
}
}
Box<Integer> intBox = new Box<>();
intBox.set(10);
int value = intBox.get();
// Generic method
public <E> void printArray(E[] array) {
for (E element : array) {
System.out.print(element + " ");
}
System.out.println();
}
Integer[] intArray = {1, 2, 3, 4, 5};
printArray(intArray);
15. Lambda Expressions and Functional Interfaces
Java 8 introduced lambda expressions and functional interfaces:
// Functional interface
@FunctionalInterface
interface MathOperation {
int operate(int a, int b);
}
// Lambda expression
MathOperation addition = (a, b) -> a + b;
System.out.println("10 + 5 = " + addition.operate(10, 5));
// Using lambda with built-in functional interfaces
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
16. Streams API
The Streams API provides a functional approach to processing collections:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Using streams to filter, map, and collect
List<Integer> evenSquares = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.collect(Collectors.toList());
System.out.println(evenSquares); // [4, 16, 36, 64, 100]
// Parallel streams for concurrent processing
long count = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.count();
System.out.println("Number of even numbers: " + count);
17. Java Module System
Introduced in Java 9, the module system helps create more maintainable and scalable applications:
// module-info.java
module com.example.myapp {
requires java.sql;
exports com.example.myapp.api;
}
18. Best Practices and Coding Standards
- Follow naming conventions: CamelCase for classes, lowerCamelCase for methods and variables
- Use meaningful and descriptive names for classes, methods, and variables
- Keep methods small and focused on a single task
- Use appropriate access modifiers to encapsulate data
- Handle exceptions properly and avoid catching generic exceptions
- Use StringBuilder for string concatenation in loops
- Prefer composition over inheritance when possible
- Use interfaces to define contracts and promote loose coupling
- Follow the DRY (Don't Repeat Yourself) principle
- Write unit tests for your code
- Use logging instead of System.out.println() for debugging
- Always close resources (files, database connections) in a finally block or use try-with-resources
- Use appropriate collection types based on your use case
- Avoid using raw types with generics
- Use stream API and lambda expressions for more readable and concise code
19. Common Interview Questions
- Q: What is the difference between an abstract class and an interface?
A: An abstract class can have both abstract and non-abstract methods, while an interface (prior to Java 8) can only have abstract methods. Abstract classes can have instance variables, while interfaces can only have static final variables. A class can implement multiple interfaces but can extend only one abstract class. - Q: Explain the difference between '==' and '.equals()' method in Java.
A: '==' compares object references (checks if two references point to the same object in memory), while '.equals()' compares the contents of objects. For primitive types, '==' compares values. - Q: What is the difference between checked and unchecked exceptions?
A: Checked exceptions are checked at compile-time and must be either caught or declared in the method signature. Unchecked exceptions (RuntimeExceptions) are not checked at compile-time and don't need to be explicitly caught or declared. - Q: Explain the concept of method overloading and method overriding.
A: Method overloading involves multiple methods in the same class with the same name but different parameters. Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. - Q: What is the purpose of the 'final' keyword in Java?
A: The 'final' keyword can be used with variables (to make them constants), methods (to prevent overriding), and classes (to prevent inheritance). - Q: Explain the difference between ArrayList and LinkedList.
A: ArrayList uses a dynamic array to store elements and provides fast random access. LinkedList uses a doubly-linked list and provides fast insertion and deletion at both ends. - Q: What is the Java Memory Model? Explain heap and stack memory.
A: The Java Memory Model specifies how the Java virtual machine works with the computer's memory. Heap memory is used for dynamic memory allocation for Java objects. Stack memory is used for static memory allocation and the execution of a thread. - Q: What is the purpose of the 'synchronized' keyword?
A: The 'synchronized' keyword is used to create thread-safe code by ensuring that only one thread can access the synchronized code block at a time. - Q: Explain the concept of Java's garbage collection.
A: Garbage collection is the process by which Java automatically frees memory occupied by objects that are no longer in use. It helps prevent memory leaks and makes memory management easier for developers. - Q: What are functional interfaces in Java 8?
A: Functional interfaces are interfaces that contain exactly one abstract method. They can have multiple default or static methods. Functional interfaces are the basis for lambda expressions in Java 8.
20. Conclusion
Java continues to be one of the most popular programming languages in the world, widely used for developing enterprise applications, Android apps, web services, and more. Its platform independence, robust standard library, and continuous evolution make it a versatile choice for developers.
This guide has covered the fundamentals of Java programming, from basic syntax to advanced concepts like multithreading and the module system. However, mastering Java requires practice, hands-on experience, and staying updated with the latest features and best practices.
As you progress in your Java journey, consider exploring more advanced topics such as:
- Design patterns and architectural principles
- Java Enterprise Edition (Java EE) and Spring Framework
- Reactive programming with Project Reactor or RxJava
- Microservices architecture
- Java persistence and ORM frameworks like Hibernate
- Testing frameworks like JUnit and Mockito
- Build tools like Maven and Gradle
- Continuous Integration and Continuous Deployment (CI/CD) for Java applications
Remember that good programming is not just about knowing the language features, but also about writing clean, efficient, and maintainable code. Continue to practice, read other developers' code, contribute to open-source projects, and stay engaged with the Java community to enhance your skills and keep up with the evolving Java ecosystem.
Keywords
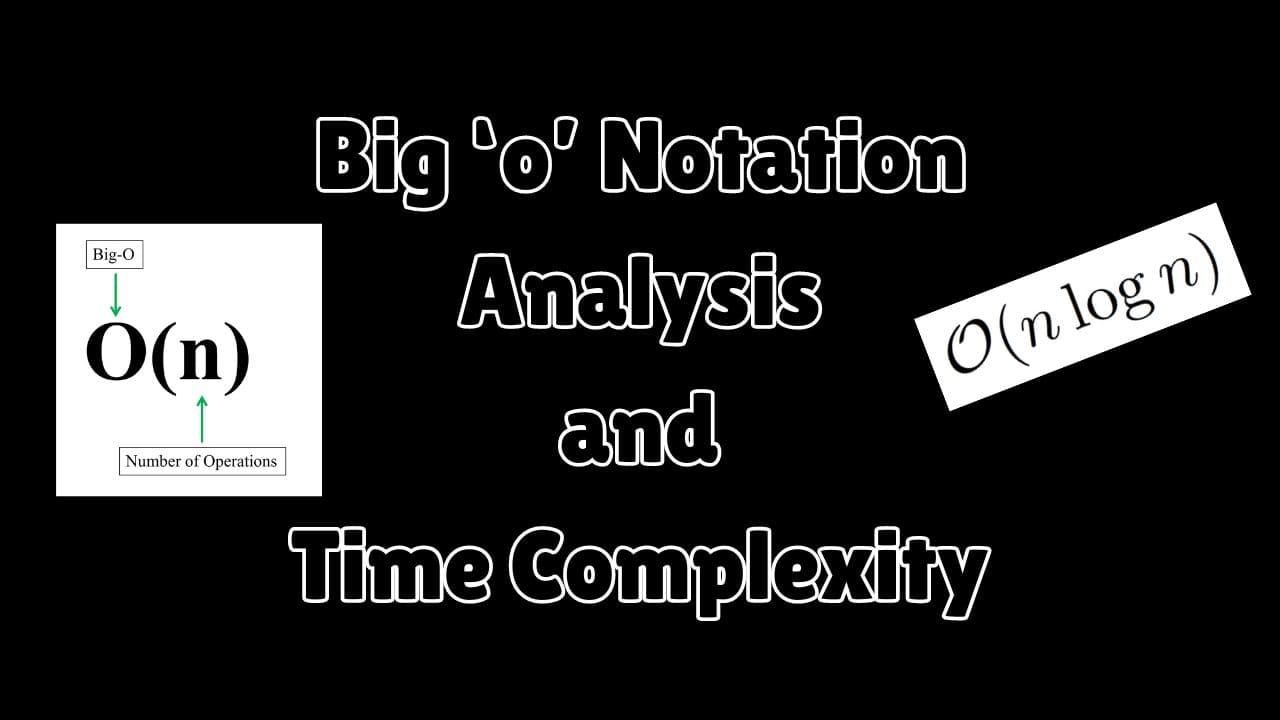
Big O Notation : explain the significance of big o notation in algorithm analysis
Big O notation is crucial in computer science for analyzing algorithm efficiency. It simplifies performance comparison, scalability analysis, and optimization guidance. Understanding time complexities
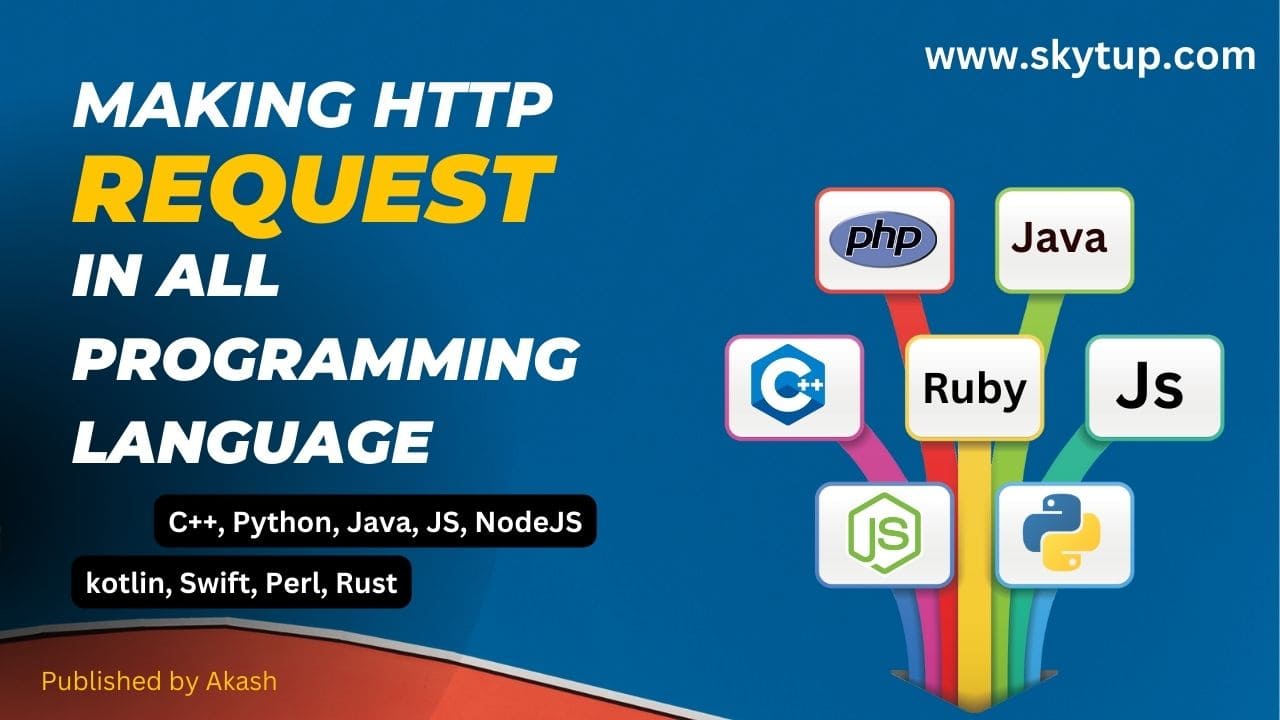
How to Make HTTP Requests in Multiple Programming Languages: JavaScript, Python, Java, Node Js, Php, Android and More
Learn how to make HTTP requests in multiple programming languages including JavaScript, Node.js, C++, Python, PHP, Ruby, Java, Go, Android Studio (Java), Swift (iOS), Perl, Rust, and Kotlin. This comp
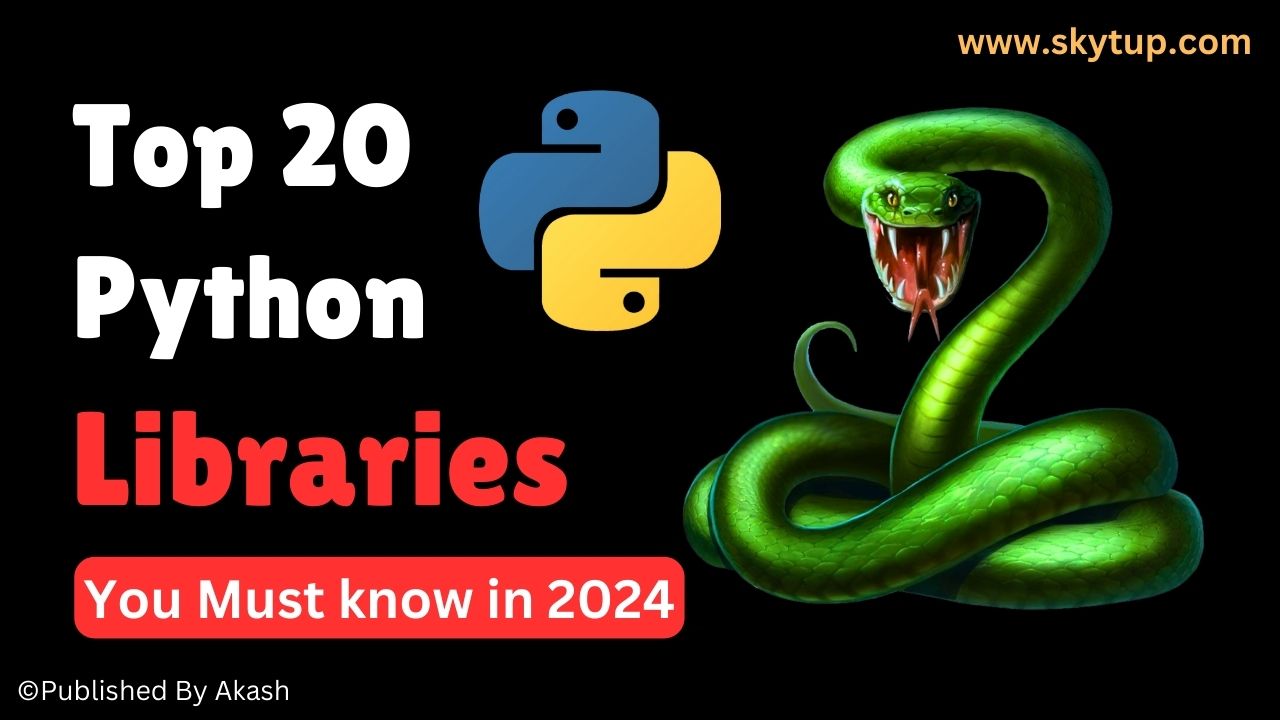
Top 20 Python Libraries You Must Know in 2024 for Data Science, Web Development, and More
Discover the top 20 Python libraries essential for 2024, spanning data science, machine learning, web development, and automation. From NumPy and Pandas to TensorFlow and Flask, these libraries are cr
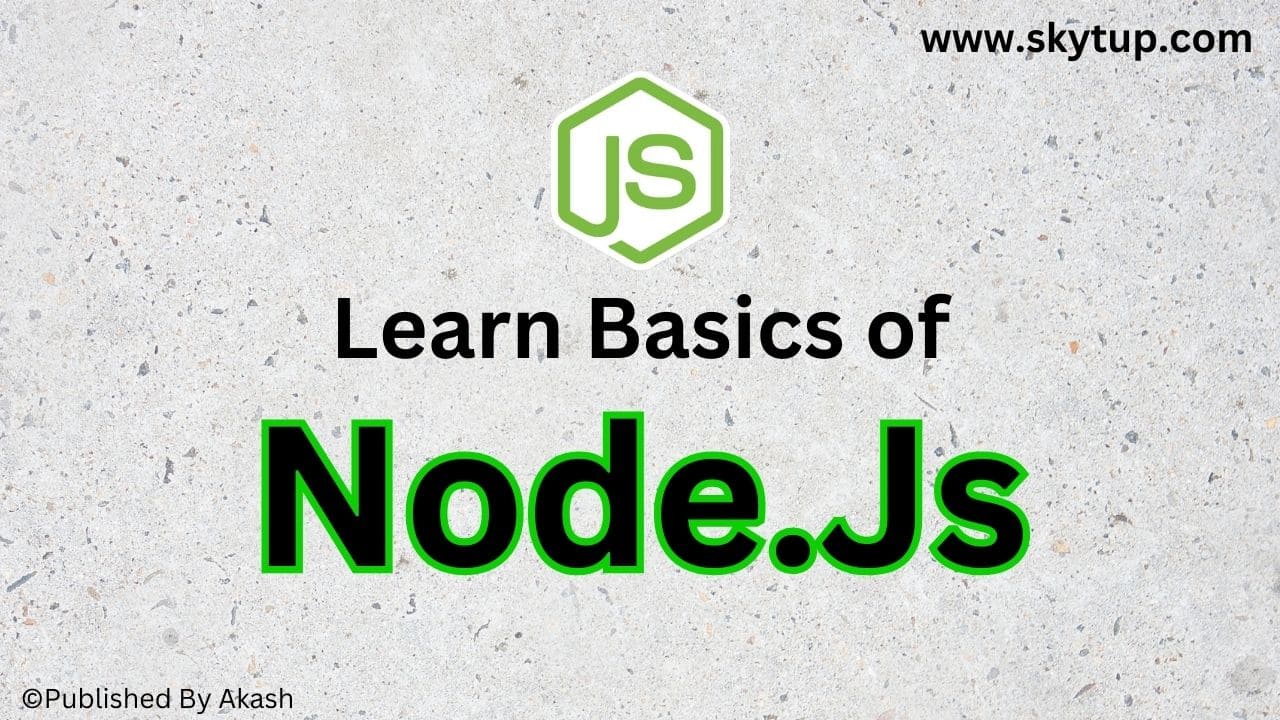
Learn Node JS from scratch | Powerful Backend Development π₯
Node.js is an open-source and cross-platform JavaScript runtime environment. It is a popular tool for almost any kind of project!
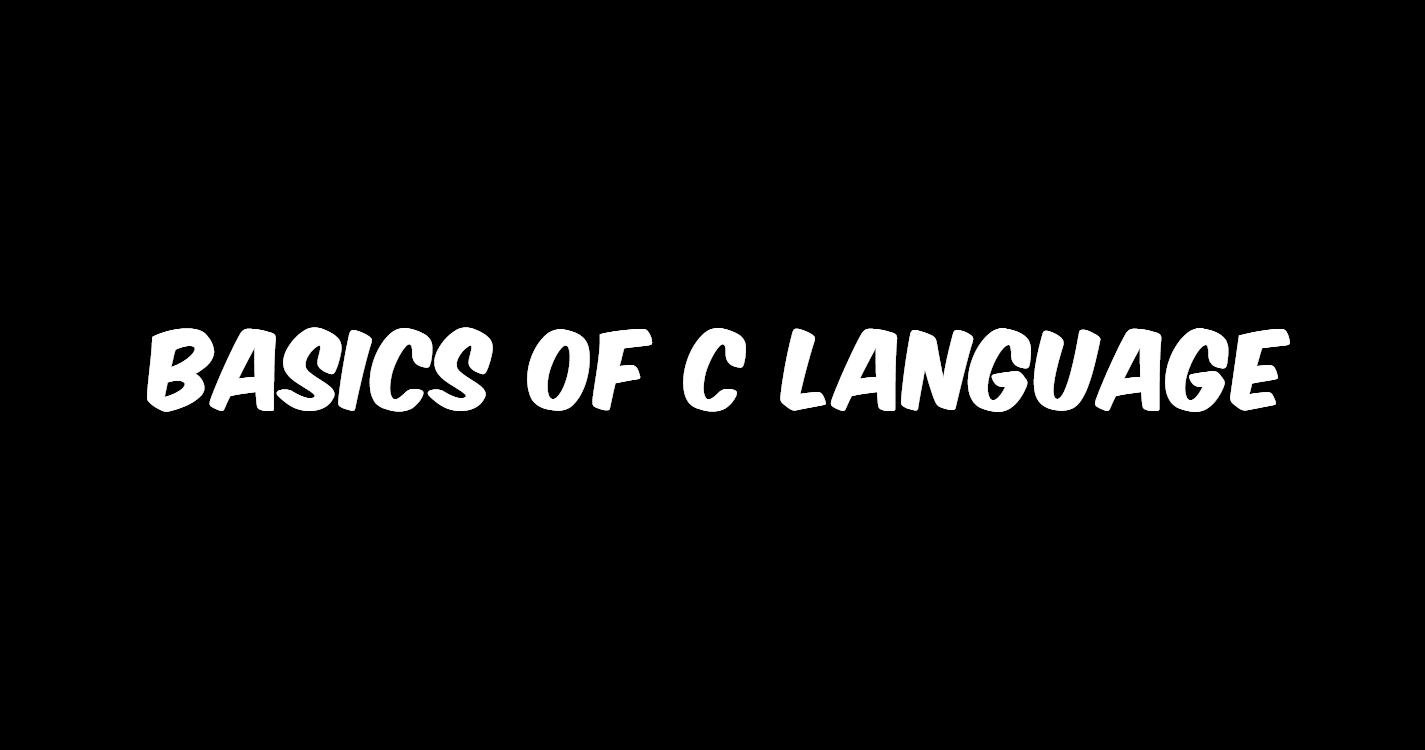
Basics of C Programming Language for Absolute Beginners | Is C Language Worth it to learn in 2024?
Learn C programming basics, syntax, and scope with our comprehensive guide. Discover why C is an essential language to learn and start your programming journey today. Get ready to unlock the power of
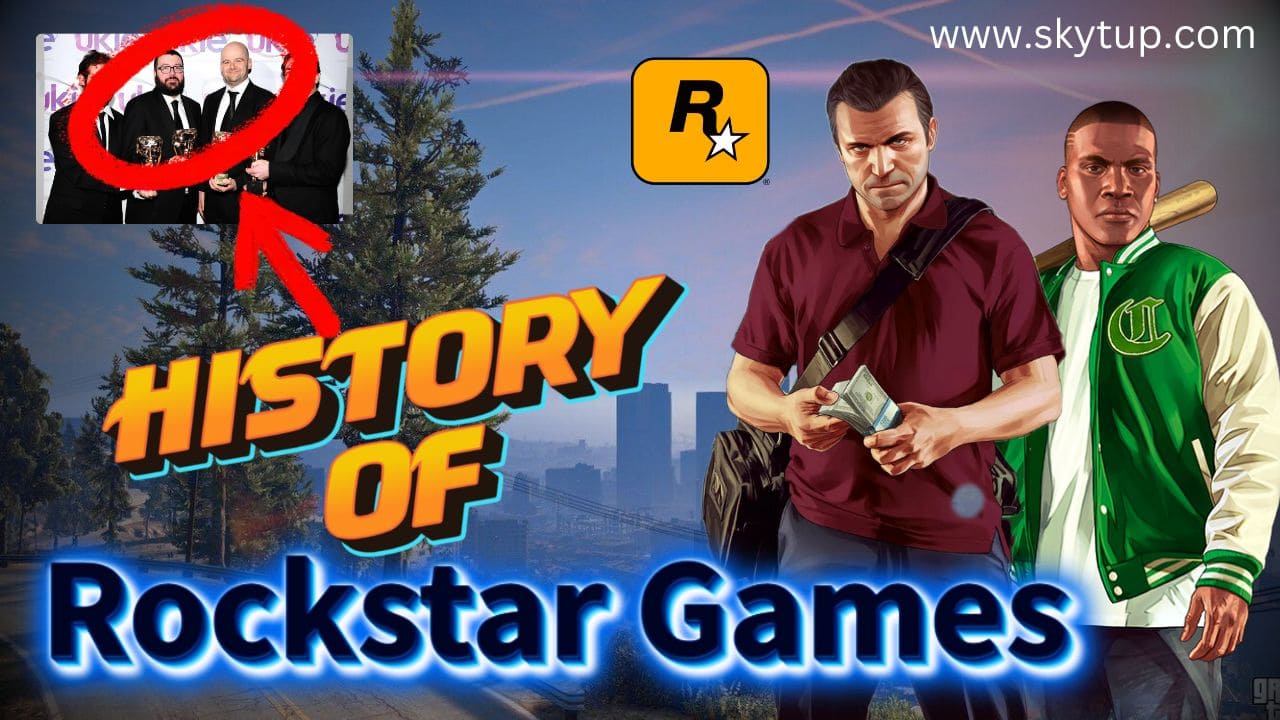
History of rockstar games in order | GTA V, GTA San Andrews, GTA Vice City, GTA IV
Rockstar Games, Inc. is an American video game publisher based in New York City. The company was established in December 1998 as a subsidiary of Take-Two Interactive, using the assets Take-Two had pre
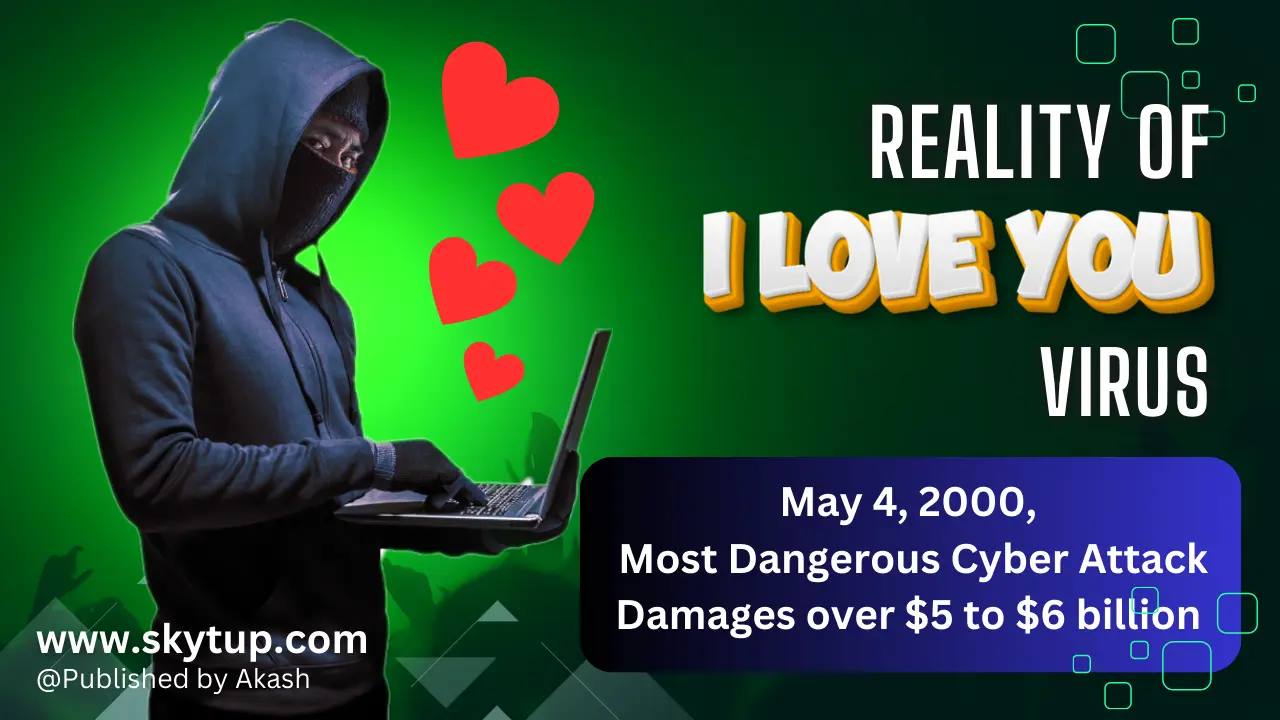
The I LOVE YOU Virus : A Historic Cyber Threat and How to Protect Yourself Today | Source Code
Discover the story behind the infamous ILOVEYOU virus, which caused billions in damages by exploiting email systems. Learn how this attack changed cybersecurity forever and get essential tips to prote
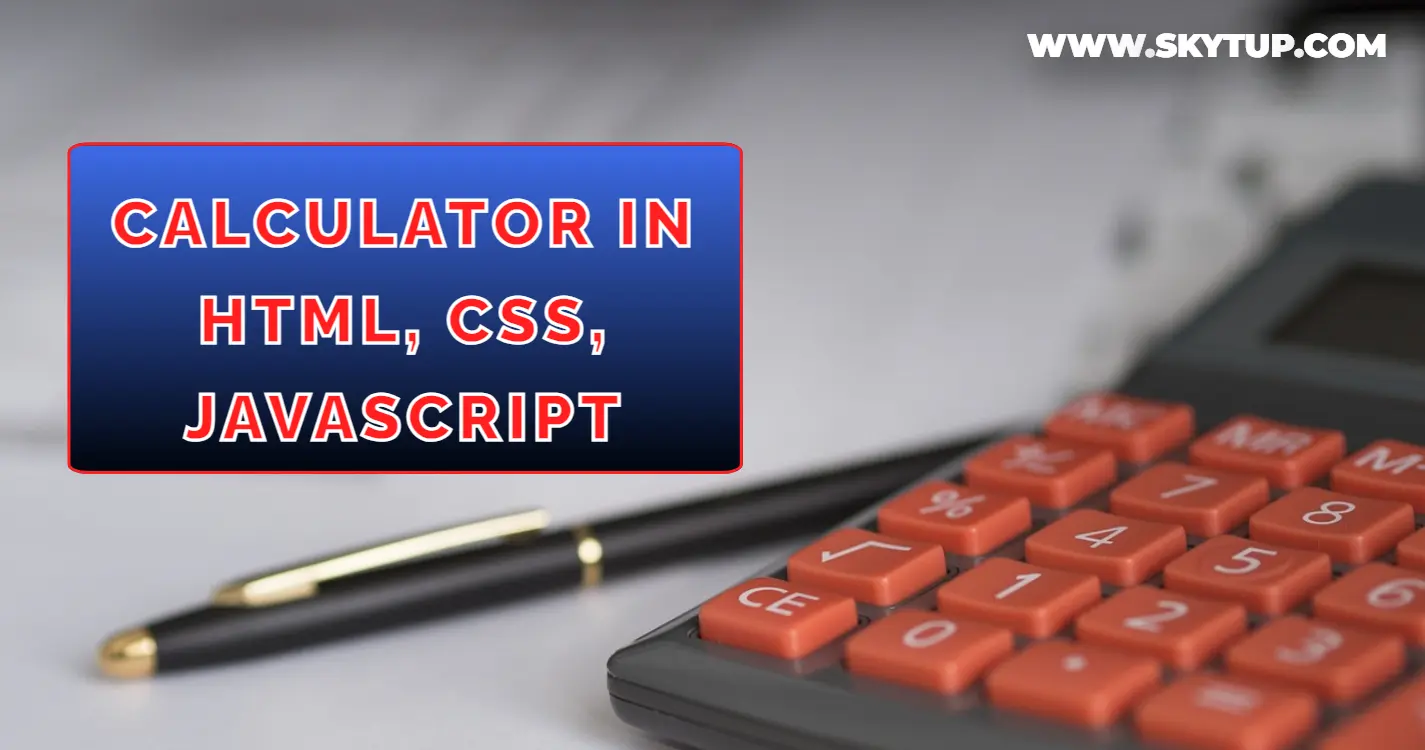
Lets Make a Simple Calculator using Html, Css, JavaScript | Source Code β
Creating an HTML Calculator using HTML, CSS, and JS Build a basic calculator using HTML, CSS, and JavaScript. Create the calculator&amp;#039;s layout with HTML, style it with CSS, and add func
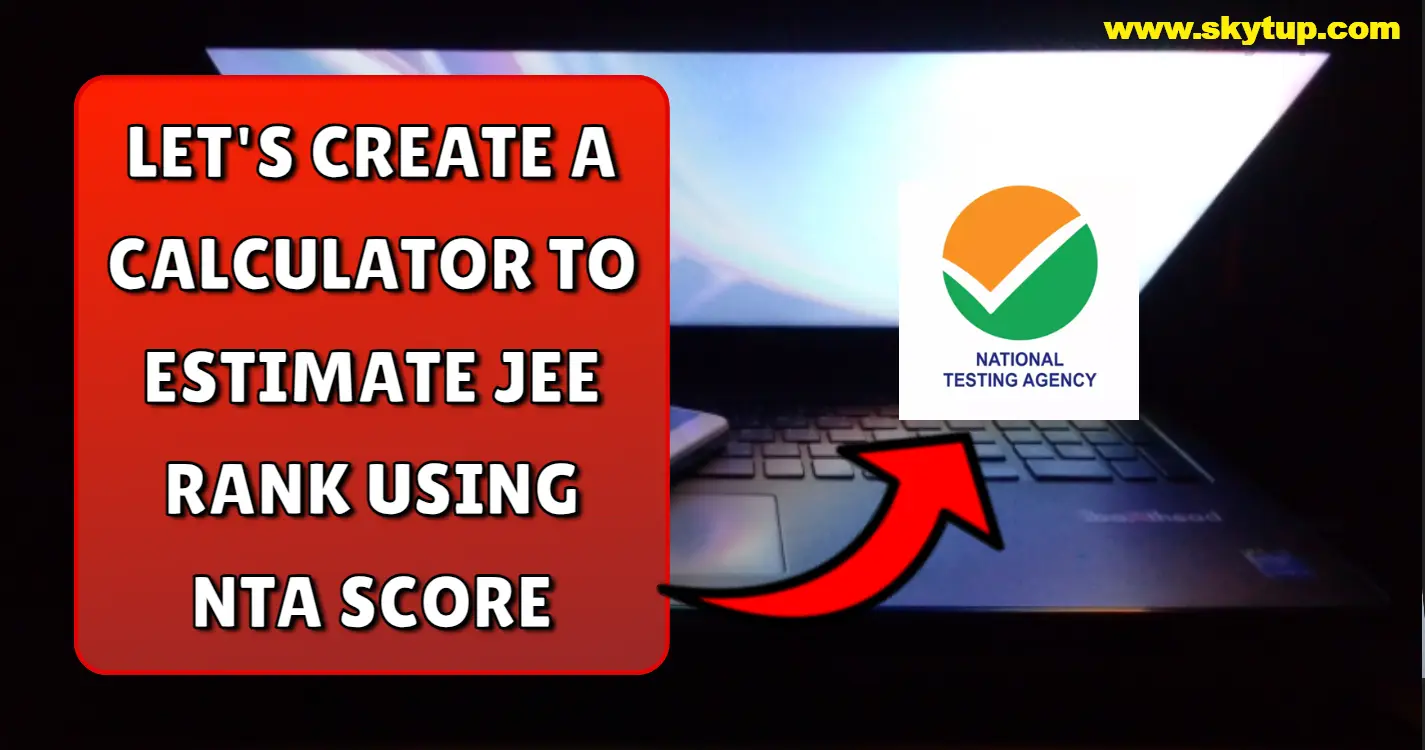
Lets Make a JEE Rank Calculator: Estimate Your 2024 JEE Mains Rank with HTML, CSS, and JavaScript
Discover how to build a JEE Rank Calculator using HTML, CSS, and JavaScript. This step-by-step guide helps aspiring engineers estimate their JEE Mains 2024 rank based on NTA scores. Learn to create an
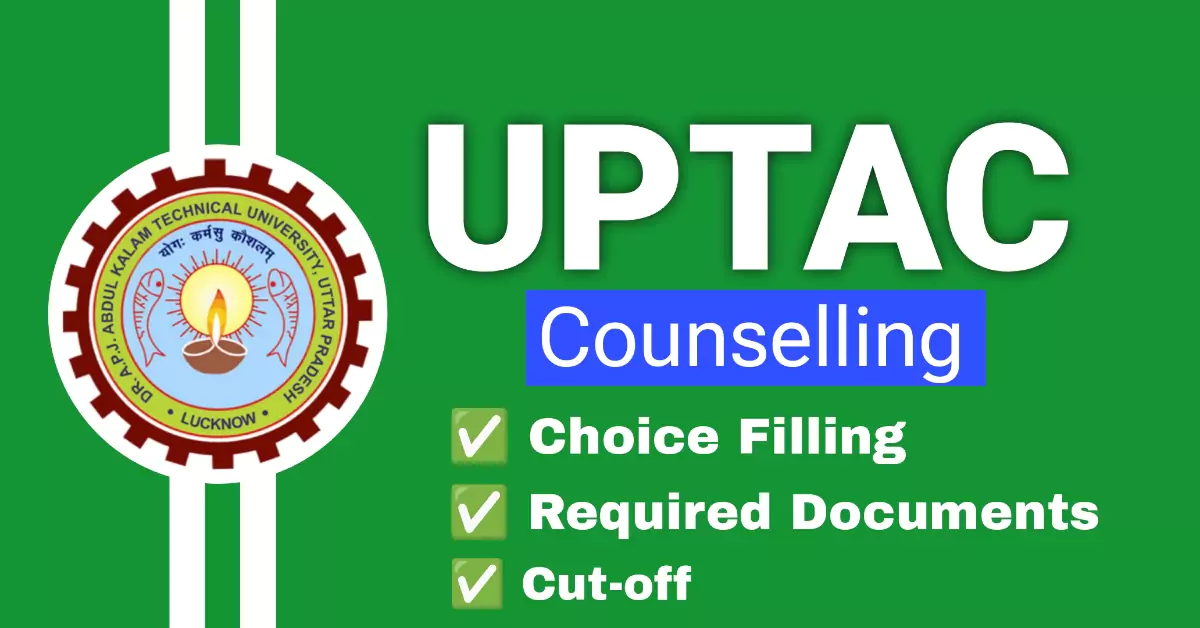
AKTU Counselling Choice FIlling BTECH 2024 | UPTAC Counselling Jee Mains | Engineering College 2024
This comprehensive guide explores the top 20 engineering colleges under AKTU for Computer Science and Engineering (CSE) and Information Technology (IT). Detailed information includes courses, fees, op
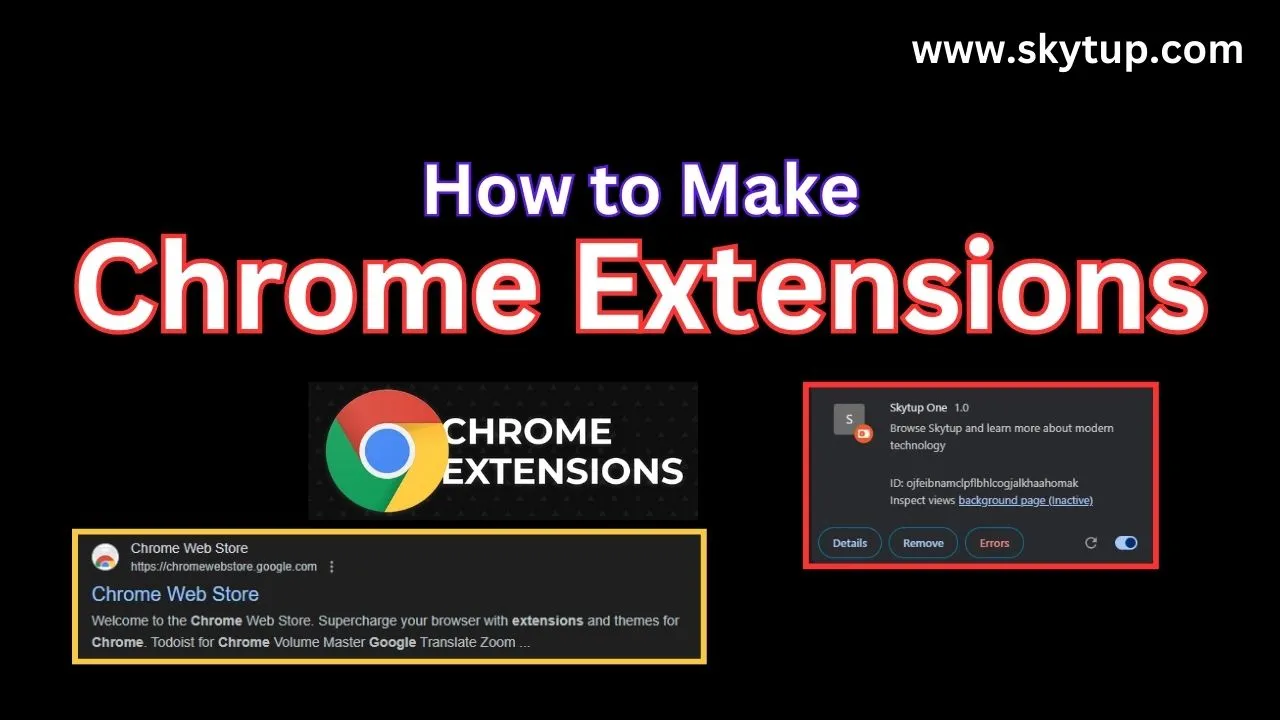
How to create google Chrome Extension in javascript for personal or Professional use
Creating Chrome extensions is a powerful way to enhance the web browsing experience and solve real-world problems. From simple UI modifications to complex integrations with web services, the possibili
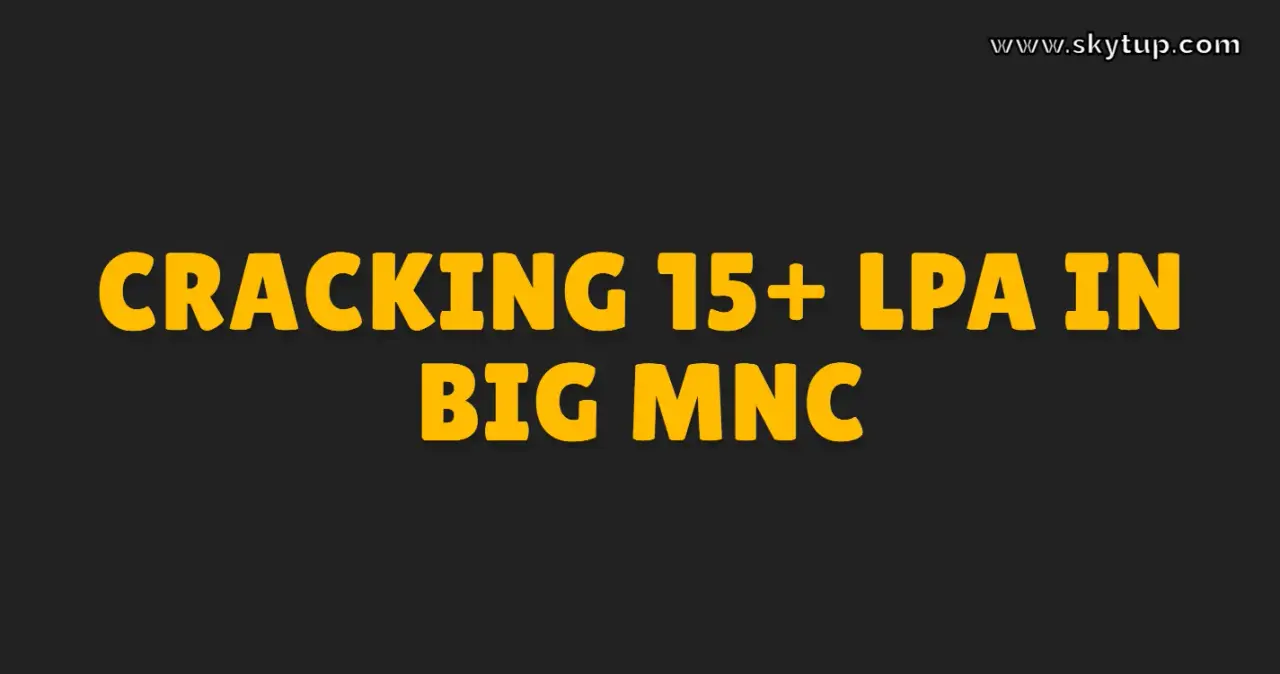
Cracking the 15+ LPA Code: Ultimate Guide to Securing Top MNC Placements
Unlock the secrets to landing high-paying jobs at leading MNCs with our comprehensive guide. From mastering data structures and algorithms to acing system design interviews, we cover it all. Learn to
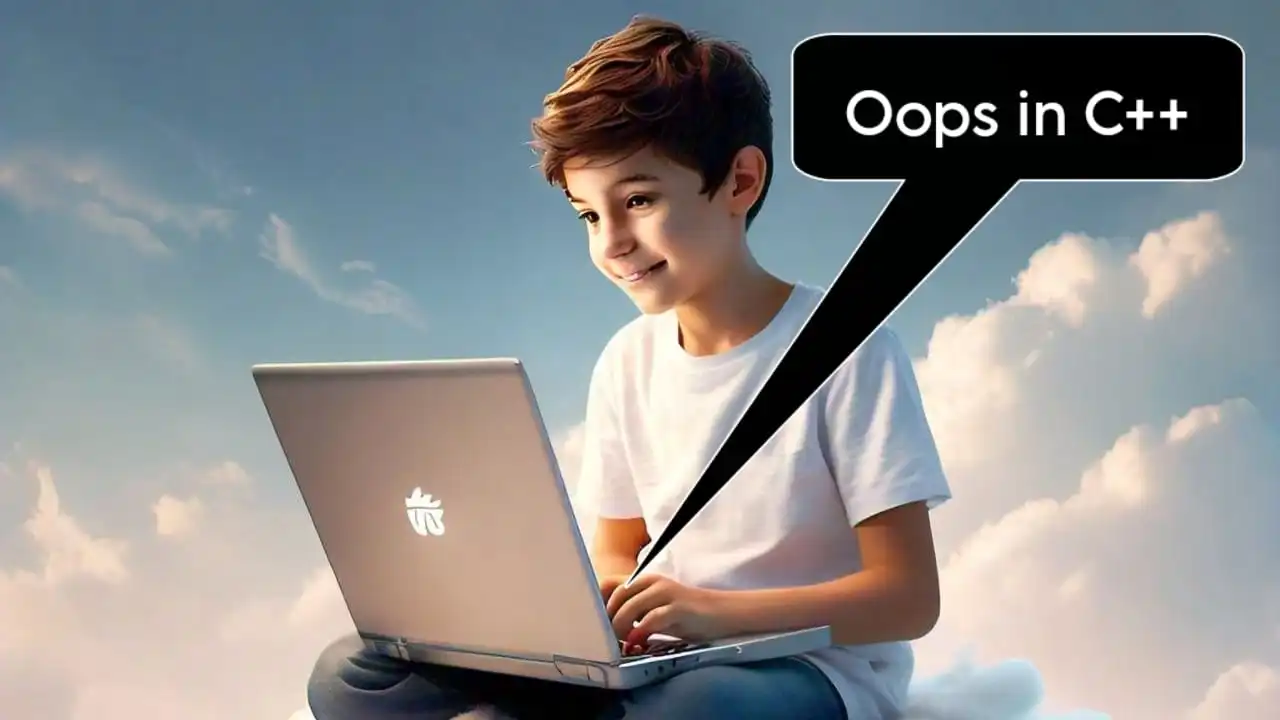
OOPs (Object Oriented Programming System) | Concepts & Interview Question with Examples
The major purpose of C++ programming is to introduce the concept of object orientation to the C programming language. Object Oriented Programming is a paradigm that provides many concepts such as inhe
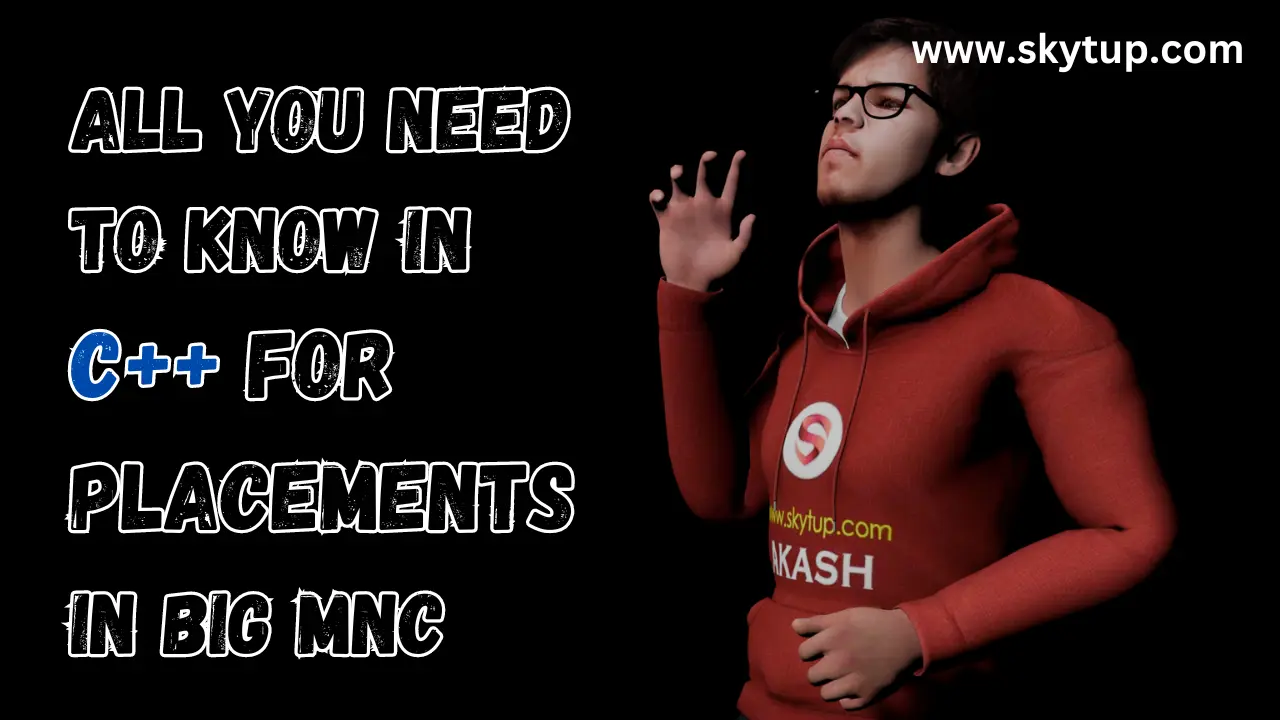
All you need to know in C++ for placement in big MNC | Requirements and eligibility criteria
Boost your chances of landing a job at top MNCs with expertise in C++. Learn the required skills, data structures, algorithms, and eligibility criteria to excel in placement tests. Stay ahead with our
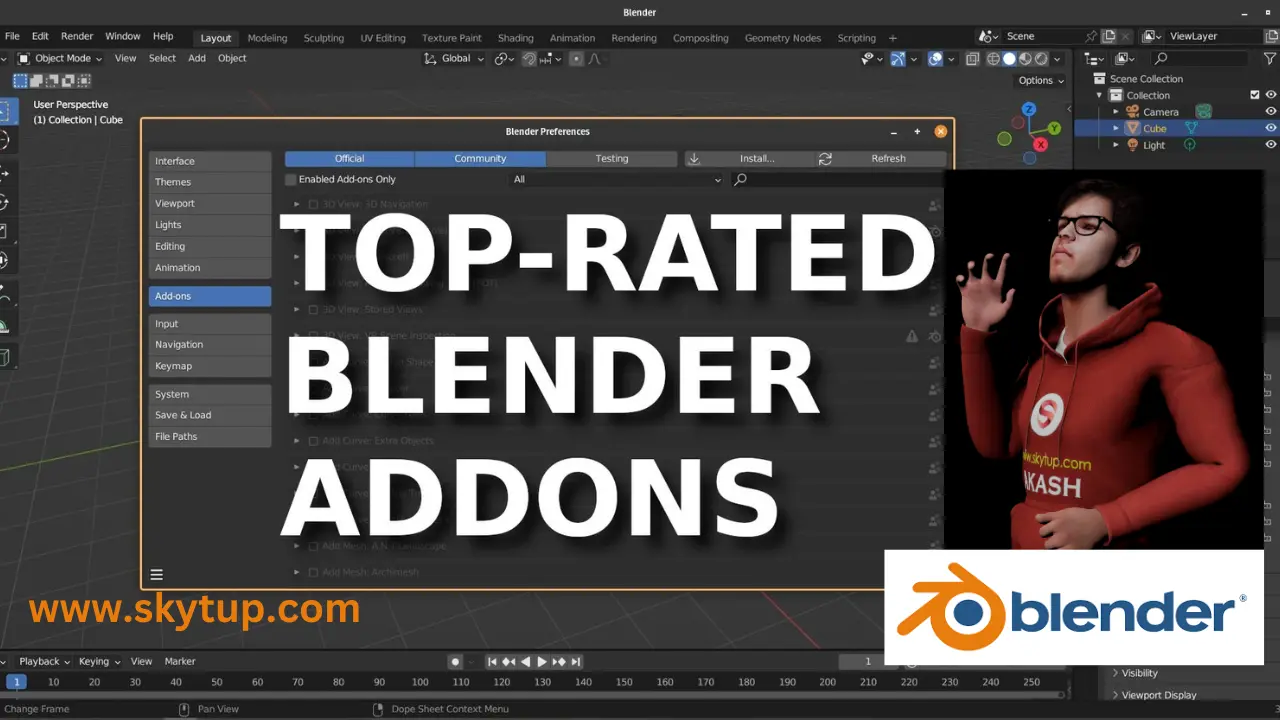
Top 15 Blender Add-ons for Becoming a Pro in the 3D World π₯
"Take your 3D skills to the next level with these top 10 Blender add-ons. From modeling and texturing to animation and rendering, discover the best tools to streamline your workflow and create s
Performance Comparison of Programming Languages: Counting from 1 to 1 Billion
This article compares the performance of various programming languages, including C, C++, Java, Python, Go, C#, and JavaScript, by measuring the time each takes to count from 1 to 1 billion. Detailed
LeetCode Problem π₯ 1945 - Sum of Digits of String After Convert
This type of problem is common in competitive programming and technical interviews, as it tests your understanding of basic string manipulation, numeric transformations, and iterative processes. In th

Building a Distance-Measuring LED Indicator Using Arduino and Ultrasonic Sensor π₯
Learn to create a distance-measuring LED indicator using an Arduino and an HC-SR04 ultrasonic sensor. This project guides you through setting up the sensor to control LEDs based on object proximity, o
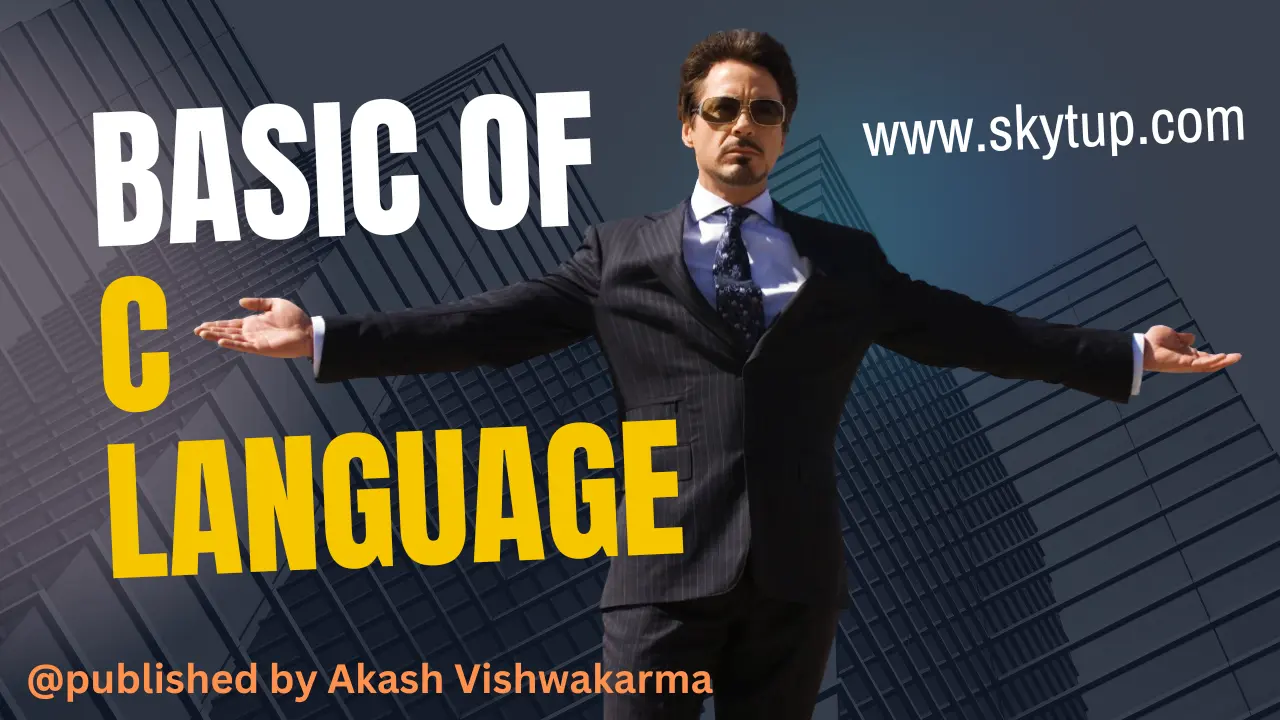
Basics of C Language for beginners | Syntax and Common Interview questionπ₯
C is a procedural programming language with a static system that has the functionality of structured programming, recursion, and lexical variable scoping. C was created with constructs that transfer w
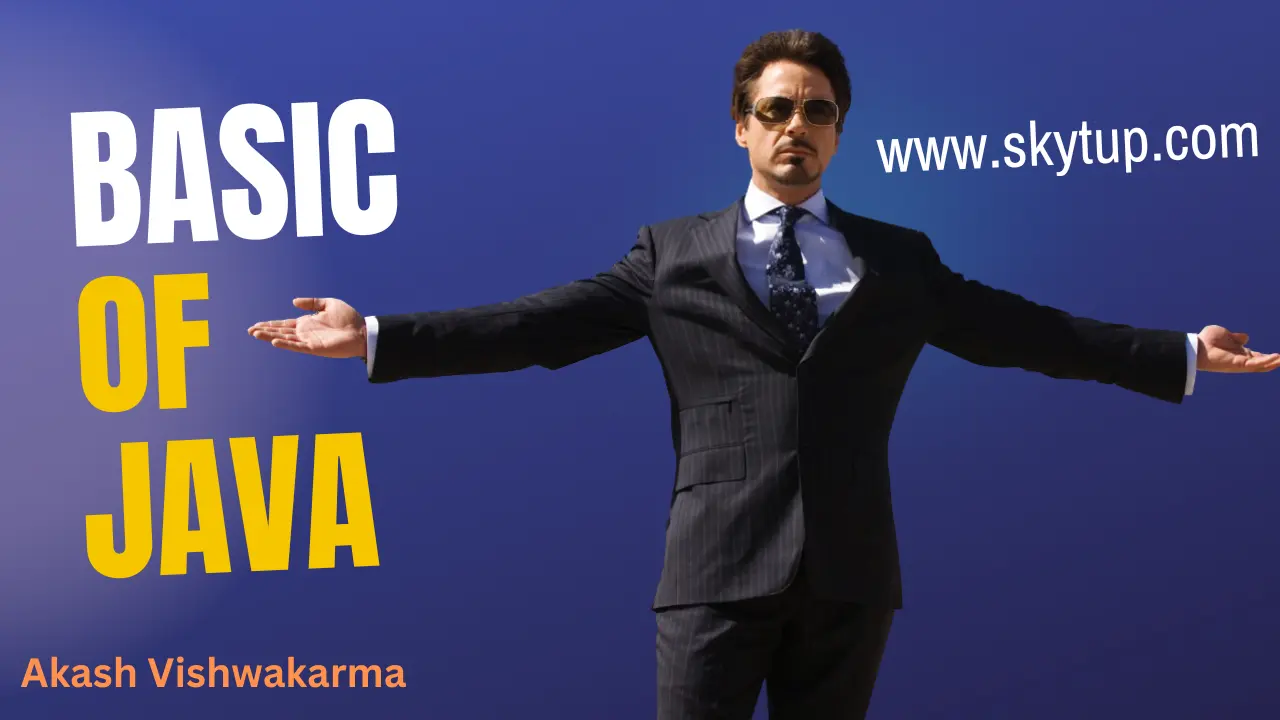
Basics of Java Programming and common Interview Questions π₯
Java Platform is a collection of programs. It helps to develop and run a program written in the Java programming language. Java Platform includes an execution engine, a compiler and set of libraries.
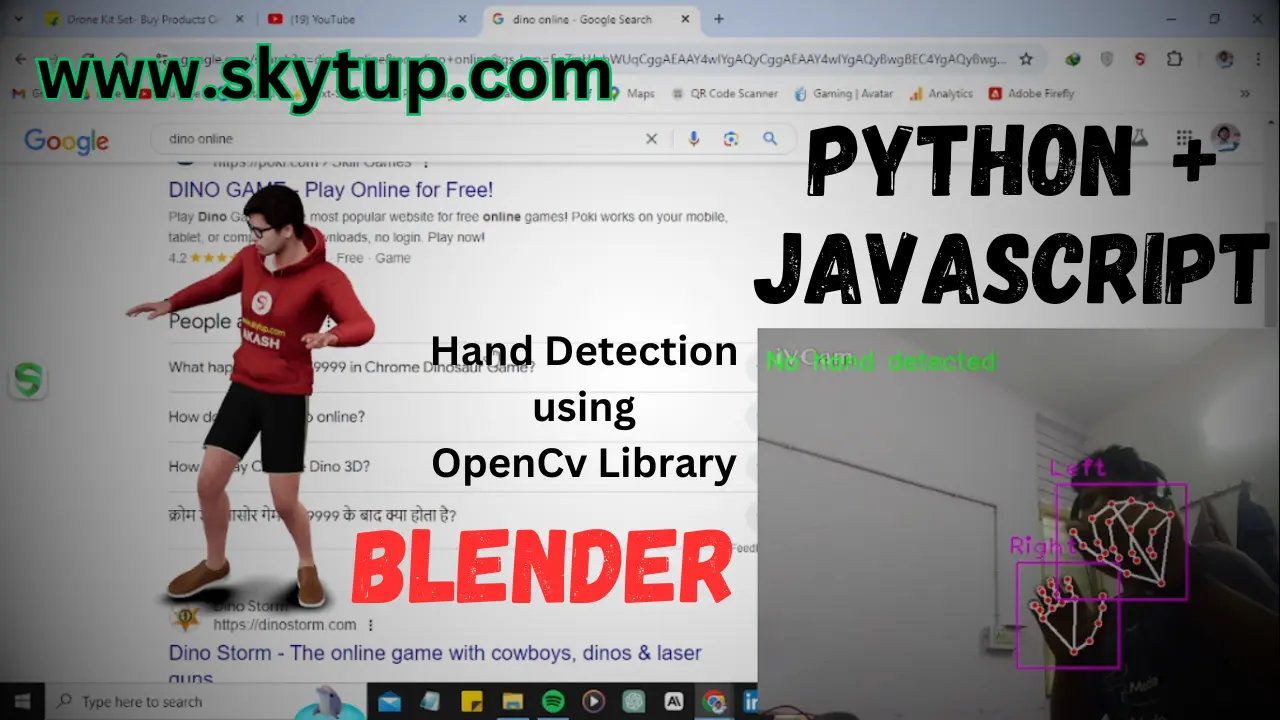
Hand Gesture Control Using OpenCV and Cvzone | Python Project by Akash Vishwakarma
Hey I am Akash Vishwakarma in this article I am gonna show you hand detection system using python with opencv library. This is an open source library which you can use to detect multiple hand gestures
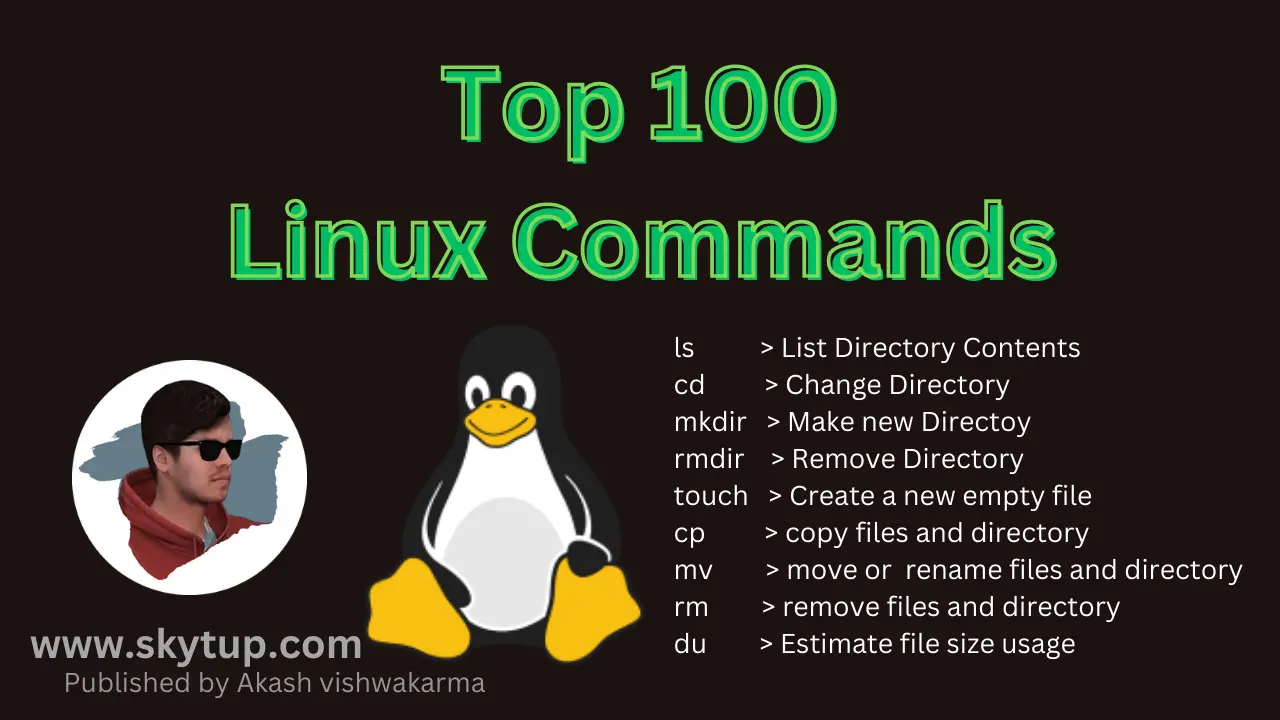
Top 100 Linux Commands you Must know in 2024 | Akash
Hi Everyone, My name is Akash in this article I you will learn top 100 linux commands. By mastering these essential Linux commands, you'll become more efficient and confident in your ability to w
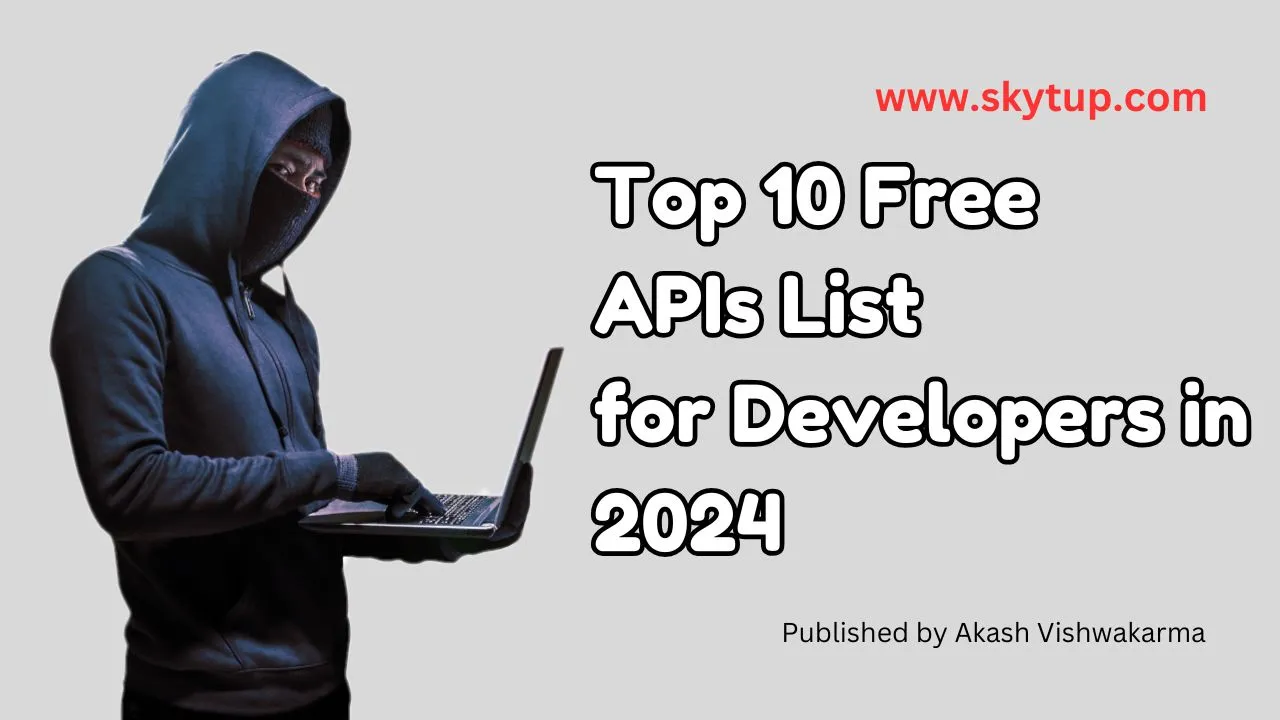
Top 10 Free APIs with Documentation for Developers in 2024 π₯
Hi Everyone this is Akash Vishwakarma. In this Article we have Top 10 Api List by using these Api Endpoints You can make Amazing Projects and showcase them on your portfolio. You can show these to rec
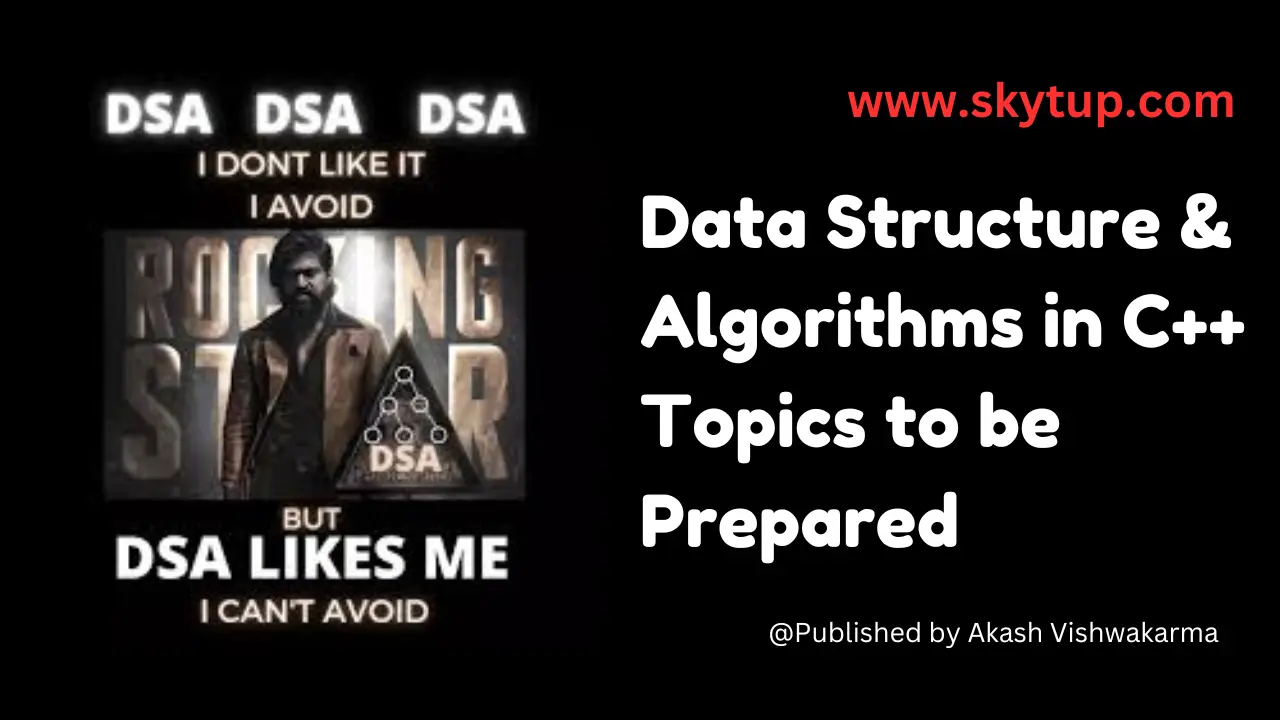
Data Structures and Algorithms in C++ | Topics to be prepared for Interviews
Hi everyone, I'm Akash Vishwakarma. As someone who passionate about programming, I've often found myself wondering what it takes to crack those tough technical interviews at top tech
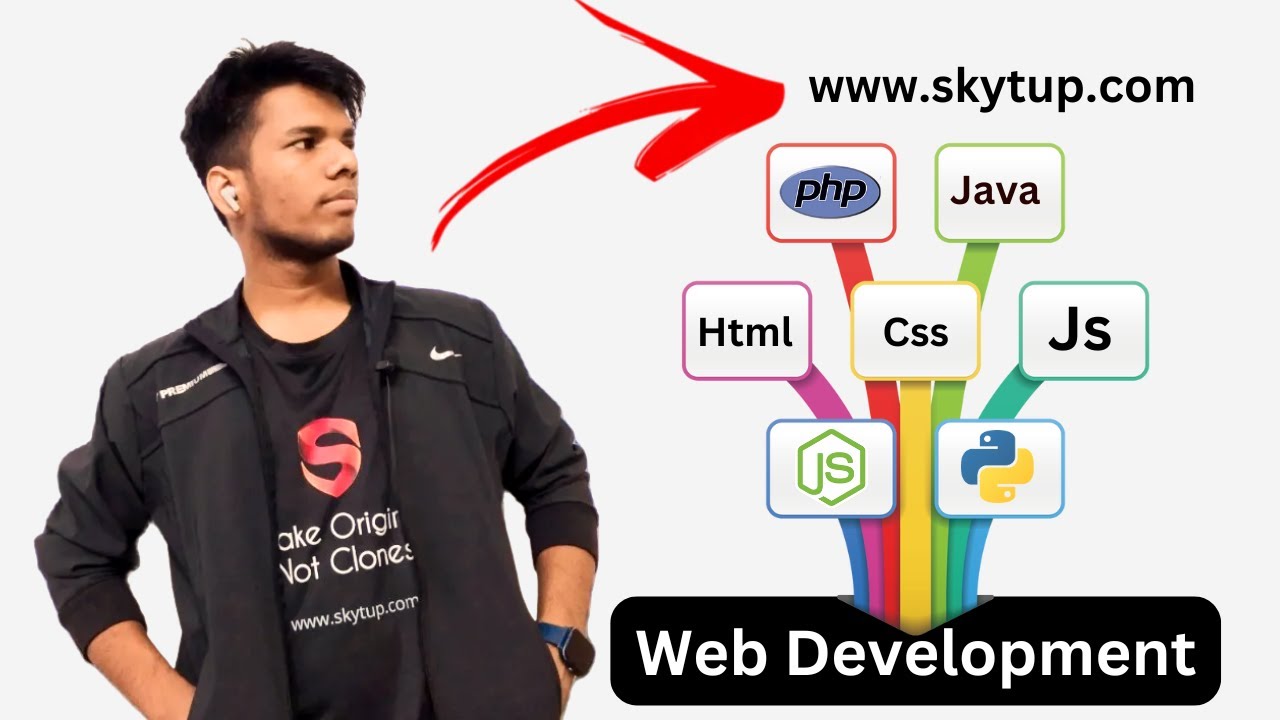
Become a Full Stack Web Developer | A Quick Roadmap for Beginners in 2025 | Developer Akash
Hi Everyone,its me Akash, In this Article I&amp;#039;ve Shared a Roadmap to become a Full Stack Web Developer. Follow guildines given in this article and you will must know something new and i

Advanced Guide to C++ STL for Competitive Programming
The C++ STL is built on three fundamental components: containers, algorithms, and iterators. Understanding how these components interact and their underlying implementations is crucial for optimal usa
#1 - Basic Fundamentals - DSA Series With C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is 1st Article of DSA Series on Skytup. In this Article We will learn about C++ Fundamentals. This article is written with the help of Ai Tools and some of my own exp
#2 - Arrays and Strings - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #2 of Our DSA Series on Skytup. In this Article We will learn about C++ Fundamentals. This article is written with the help of Ai Tools and some of my o
#3 - Linked Lists - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #3 of Our DSA Series on Skytup. In this Article We will learn about Linked Lists. This article is written with the help of Ai Tools and some of my own e
#4 - Stacks and Queues - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #4 of Our DSA Series on Skytup. In this Article We will learn about C++ Stack and Queues. This article is written with the help of Ai Tools and some of
#5 - Trees - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #5 of Our DSA Series on Skytup. In this Article We will learn about C++ Trees. This article is written with the help of Ai Tools and some of my own expe