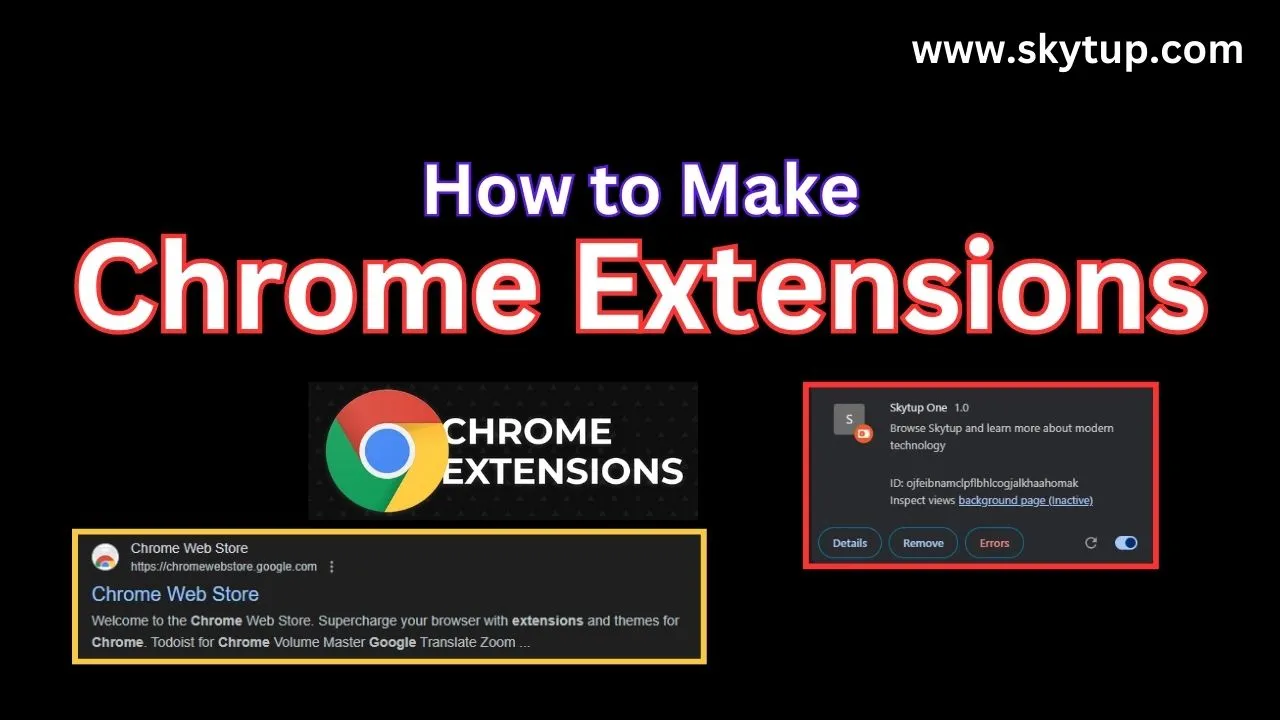
How to create google Chrome Extension in javascript for personal or Professional use
Creating Your Own Chrome Extension
In the ever-evolving landscape of web development, Chrome extensions have emerged as powerful tools that enhance browser functionality and user experience. This comprehensive guide will walk you through the intricacies of creating Chrome extensions, from basic concepts to advanced techniques. Whether you're a seasoned developer or a curious beginner, this article will equip you with the knowledge and skills to build your own Chrome extensions.
1. Understanding Chrome Extensions
Chrome extensions are small software programs that customize the browsing experience. They're built using web technologies such as HTML, CSS, and JavaScript, making them accessible to a wide range of developers. Extensions can modify and enhance the functionality of the Chrome browser, allowing users to tailor their browsing experience to their specific needs.
1.1 Key Components of a Chrome Extension
- Manifest File: The heart of every Chrome extension, defining its properties and capabilities.
- Background Scripts: JavaScript files that run in the background and manage the extension's behavior.
- Content Scripts: JavaScript files that can be injected into web pages to interact with their content.
- Popup: An HTML file that creates a user interface when the extension icon is clicked.
- Options Page: An HTML page that allows users to customize the extension's settings.
1.2 Chrome Extension Capabilities
Chrome extensions can perform a wide variety of tasks, including:
- Modifying web page content
- Adding custom CSS styles to pages
- Implementing keyboard shortcuts
- Creating custom right-click context menu options
- Interacting with browser tabs and windows
- Making network requests
- Storing and retrieving data
2. Setting Up Your Development Environment
Before diving into extension development, it's crucial to set up a proper development environment. Here's what you'll need:
- Text Editor or IDE: Choose a code editor you're comfortable with. Popular options include Visual Studio Code, Sublime Text, or WebStorm.
- Google Chrome: Ensure you have the latest version of Google Chrome installed.
- Version Control: Use Git for version control and GitHub for repository hosting.
- Node.js and npm: While not strictly necessary, these tools can be helpful for managing dependencies and build processes.
3. Creating Your First Chrome Extension
Let's start by creating a simple Chrome extension that changes the background color of web pages. We'll go through each step in detail.
3.1 Creating the Manifest File
First, create a new directory for your extension project. Inside this directory, create a file named manifest.json
. This file is crucial as it provides important information about your extension to Chrome.
{
"manifest_version": 3,
"name": "Background Color Changer",
"version": "1.0",
"description": "Changes the background color of web pages",
"permissions": ["activeTab"],
"action": {
"default_popup": "popup.html",
"default_icon": {
"16": "images/icon16.png",
"48": "images/icon48.png",
"128": "images/icon128.png"
}
},
"icons": {
"16": "images/icon16.png",
"48": "images/icon48.png",
"128": "images/icon128.png"
}
}
This manifest file defines the basic structure of your extension. Let's break down its components:
manifest_version
: Specifies the version of the manifest file format (use 3 for the latest version).name
andversion
: Identify your extension.description
: A brief explanation of what your extension does.permissions
: Declares the permissions your extension needs (in this case, access to the active tab).action
: Defines the extension's icon and popup.icons
: Specifies the icons used for the extension in various contexts.
3.2 Creating the Popup
Next, create a file named popup.html
in your project directory. This will be the user interface for your extension when users click its icon.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Background Color Changer</title>
<style>
body {
width: 300px;
padding: 10px;
font-family: Arial, sans-serif;
}
button {
margin-top: 10px;
padding: 5px 10px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
</style>
</head>
<body>
<h1>Background Color Changer</h1>
<p>Click the button to change the background color of the current page.</p>
<button id="changeColor">Change Color</button>
<script src="popup.js"></script>
</body>
</html>
3.3 Adding Functionality with JavaScript
Create a file named popup.js
to add interactivity to your extension:
document.addEventListener('DOMContentLoaded', function() {
var changeColorButton = document.getElementById('changeColor');
changeColorButton.addEventListener('click', function() {
chrome.tabs.query({active: true, currentWindow: true}, function(tabs) {
chrome.tabs.executeScript(
tabs[0].id,
{code: 'document.body.style.backgroundColor = "#" + Math.floor(Math.random()*16777215).toString(16);'}
);
});
});
});
This script adds a click event listener to the button. When clicked, it changes the background color of the active tab to a random color.
3.4 Adding Icons
Create an images
folder in your project directory and add icon images in sizes 16x16, 48x48, and 128x128 pixels. These icons will be used in various places in the Chrome UI.
3.5 Loading Your Extension
Now that you have created all the necessary files, it's time to load your extension into Chrome:
- Open Google Chrome and navigate to
chrome://extensions
. - Enable "Developer mode" by toggling the switch in the top right corner.
- Click on "Load unpacked" and select your extension directory.
Your extension should now appear in Chrome's toolbar!
4. Advanced Chrome Extension Development
Now that we've covered the basics, let's delve into more advanced topics in Chrome extension development.
4.1 Background Scripts
Background scripts run in the background and manage the extension's behavior. They can listen for browser events and react accordingly. To add a background script, update your manifest.json
:
{
...
"background": {
"service_worker": "background.js"
},
...
}
Then create a background.js
file:
chrome.runtime.onInstalled.addListener(function() {
console.log('Extension installed');
});
chrome.tabs.onUpdated.addListener(function(tabId, changeInfo, tab) {
if (changeInfo.status === 'complete' && tab.url.indexOf('http') === 0) {
chrome.tabs.sendMessage(tabId, {action: "updateDOM"});
}
});
This background script logs a message when the extension is installed and sends a message to content scripts when a tab is updated.
4.2 Content Scripts
Content scripts are JavaScript files that run in the context of web pages. They can read and modify the DOM of web pages the user visits. To add a content script, update your manifest.json
:
{
...
"content_scripts": [
{
"matches": [""],
"js": ["content.js"]
}
],
...
}
Then create a content.js
file:
chrome.runtime.onMessage.addListener(function(request, sender, sendResponse) {
if (request.action === "updateDOM") {
document.body.style.border = "5px solid red";
}
});
This content script listens for messages from the background script and modifies the DOM when it receives an "updateDOM" message.
4.3 Chrome Storage API
Chrome provides a Storage API that allows extensions to save and retrieve data. This is useful for persisting user preferences or extension state. To use storage, add the "storage" permission to your manifest:
{
...
"permissions": ["storage"],
...
}
Then you can use the storage API in your scripts:
// Save data
chrome.storage.sync.set({key: value}, function() {
console.log('Value is set to ' + value);
});
// Retrieve data
chrome.storage.sync.get(['key'], function(result) {
console.log('Value currently is ' + result.key);
});
4.4 Cross-Origin XMLHttpRequest
Chrome extensions can make cross-origin requests, which is useful for interacting with web APIs. Add the necessary permissions to your manifest:
{
...
"permissions": [""],
...
}
Then you can make XMLHttpRequests in your scripts:
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://api.example.com/data", true);
xhr.onreadystatechange = function() {
if (xhr.readyState == 4) {
var resp = JSON.parse(xhr.responseText);
console.log(resp);
}
}
xhr.send();
4.5 Context Menus
Chrome extensions can add items to the browser's context menu. Add the "contextMenus" permission to your manifest:
{
...
"permissions": ["contextMenus"],
...
}
Then you can create context menu items in your background script:
chrome.contextMenus.create({
id: "sampleContextMenu",
title: "Sample Context Menu",
contexts: ["all"]
});
chrome.contextMenus.onClicked.addListener(function(info, tab) {
if (info.menuItemId == "sampleContextMenu") {
console.log("Context menu clicked");
}
});
5. Best Practices for Chrome Extension Development
As you develop more complex Chrome extensions, keep these best practices in mind:
5.1 Performance Optimization
- Minimize the use of background pages and prefer event pages when possible.
- Use chrome.storage.local for large amounts of data to avoid syncing delays.
- Avoid excessive DOM manipulation in content scripts.
- Use chrome.tabs.executeScript judiciously, as it can be resource-intensive.
5.2 Security Considerations
- Use Content Security Policy (CSP) to mitigate the risk of cross-site scripting attacks.
- Be cautious when handling user data and avoid storing sensitive information.
- Use HTTPS for all network requests to ensure data integrity and privacy.
- Regularly update your extension to address any security vulnerabilities.
5.3 User Experience
- Design a clear and intuitive user interface for your extension.
- Provide helpful error messages and feedback to users.
- Offer customization options to cater to different user preferences.
- Ensure your extension is accessible and works well with screen readers.
5.4 Code Organization
- Use modules to organize your JavaScript code.
- Implement a consistent naming convention for your files and functions.
- Comment your code thoroughly to improve maintainability.
- Use version control (like Git) to track changes and collaborate with others.
6. Testing and Debugging Chrome Extensions
Thorough testing is crucial for developing reliable Chrome extensions. Here are some strategies for testing and debugging:
6.1 Manual Testing
- Test your extension on different websites to ensure compatibility.
- Verify that all features work as expected in different scenarios.
- Check for any conflicts with other extensions.
6.2 Automated Testing
- Use Jest or Mocha for unit testing your JavaScript functions.
- Implement end-to-end testing with tools like Puppeteer or Selenium.
- Set up continuous integration to run tests automatically on code changes.
6.3 Debugging Techniques
- Use
console.log()
statements to output debug information. - Leverage Chrome's built-in DevTools for debugging.
- Use the chrome.runtime.getBackgroundPage() method to access the background page console.
- Implement error handling and logging to catch and diagnose issues in production.
7. Publishing Your Chrome Extension
Once you've developed and thoroughly tested your extension, you can publish it to the Chrome Web Store:
- Create a developer account on the Chrome Web Store.
- Prepare promotional materials (screenshots, promotional images, and a detailed description).
- Package your extension into a ZIP file.
- Upload your extension package to the Chrome Web Store.
- Set pricing (if applicable) and distribution options.
- Submit your extension for review.
- Certainly. I'll continue the article from where we left off: Copy
- Prepare promotional materials (screenshots, promotional images, and a detailed description).
- Package your extension into a ZIP file.
- Upload your extension package to the Chrome Web Store.
- Set pricing (if applicable) and distribution options.
- Submit your extension for review.
The review process typically takes a few days to a couple of weeks. Once approved, your extension will be available for users to install from the Chrome Web Store.
8. Monetization Strategies
If you're interested in monetizing your Chrome extension, consider these approaches:
- Freemium Model: Offer a free version with basic features and a premium version with advanced functionality.
- One-time Purchase: Charge users a one-time fee to download and use your extension.
- Subscription Model: Offer ongoing access to your extension's features for a recurring fee.
- In-app Purchases: Provide additional features or content that users can purchase within the extension.
- Advertisements: Include non-intrusive ads in your extension (be sure to comply with Chrome Web Store policies).
Remember to clearly communicate your monetization strategy to users and provide value that justifies any costs.
9. Maintaining and Updating Your Extension
Maintaining your Chrome extension is an ongoing process. Here are some key considerations:
9.1 Regular Updates
- Keep your extension compatible with the latest versions of Chrome.
- Address bug reports and user feedback promptly.
- Implement new features and improvements based on user needs and requests.
- Stay informed about changes to Chrome's extension APIs and adjust your code accordingly.
9.2 User Support
- Monitor and respond to user reviews and ratings on the Chrome Web Store.
- Provide clear channels for users to report issues or request support (e.g., email, website, or community forum).
- Maintain documentation or a FAQ to help users understand and troubleshoot common issues.
9.3 Performance Monitoring
- Use analytics tools to track usage patterns and identify areas for improvement.
- Monitor your extension's impact on browser performance and optimize as necessary.
- Keep an eye on error logs and crash reports to quickly address any stability issues.
10. Advanced Chrome Extension Features
As you become more comfortable with Chrome extension development, you might want to explore some advanced features:
10.1 Native Messaging
Native messaging allows your extension to communicate with a native application installed on the user's computer. This can be useful for integrating with system-level functionality or existing desktop applications.
// In your extension's JavaScript
chrome.runtime.sendNativeMessage('com.example.app',
{ text: "Hello from Chrome!" },
function(response) {
console.log("Received " + response);
}
);
10.2 Chrome Alarms API
The Alarms API allows you to schedule code to run periodically or at a specified time in the future.
chrome.alarms.create("myAlarm", { periodInMinutes: 30 });
chrome.alarms.onAlarm.addListener(function(alarm) {
if (alarm.name === "myAlarm") {
console.log("Alarm triggered");
}
});
10.3 Web Accessible Resources
You can make certain resources in your extension accessible to web pages or other extensions by declaring them as web accessible resources in your manifest:
{
...
"web_accessible_resources": [
{
"resources": ["images/my-image.png"],
"matches": ["https://*.example.com/*"]
}
]
...
}
10.4 Chrome Devtools Extensions
You can create extensions that add new functionality to Chrome's DevTools. This is particularly useful for creating developer tools and debugging aids.
{
...
"devtools_page": "devtools.html"
...
}
11. Case Studies: Successful Chrome Extensions
Let's look at some popular Chrome extensions and what we can learn from them:
11.1 Grammarly
Key Takeaways:
- Seamless integration with web forms and text inputs
- Clear value proposition: improving writing quality
- Freemium model with a clear upgrade path
11.2 LastPass
Key Takeaways:
- Strong focus on security and user trust
- Cross-platform synchronization
- Integration with browser's built-in password management
11.3 Momentum
Key Takeaways:
- Beautiful, customizable new tab experience
- Integration of multiple features (todo list, weather, inspirational quotes)
- Regular updates with new features and artwork
12. Future of Chrome Extension Development
As web technologies evolve, so does Chrome extension development. Here are some trends to watch:
- Progressive Web Apps (PWAs) Integration: Closer integration between extensions and PWAs.
- Enhanced Security Measures: More stringent security policies and review processes.
- AI and Machine Learning: Increased use of AI capabilities in extensions.
- Cross-browser Compatibility: Movement towards standardization across different browsers.
- Privacy-focused Features: More tools for users to control their data and privacy.
Conclusion
Creating Chrome extensions is a powerful way to enhance the web browsing experience and solve real-world problems. From simple UI modifications to complex integrations with web services, the possibilities are vast. As you embark on your journey of Chrome extension development, remember to focus on providing value to users, maintaining security and performance, and staying up-to-date with the latest web technologies.
Whether you're building tools for productivity, entertainment, or anything in between, Chrome extensions offer a unique platform to reach millions of users worldwide. So, roll up your sleeves, fire up your code editor, and start building the next great Chrome extension!
Happy coding!
Keywords
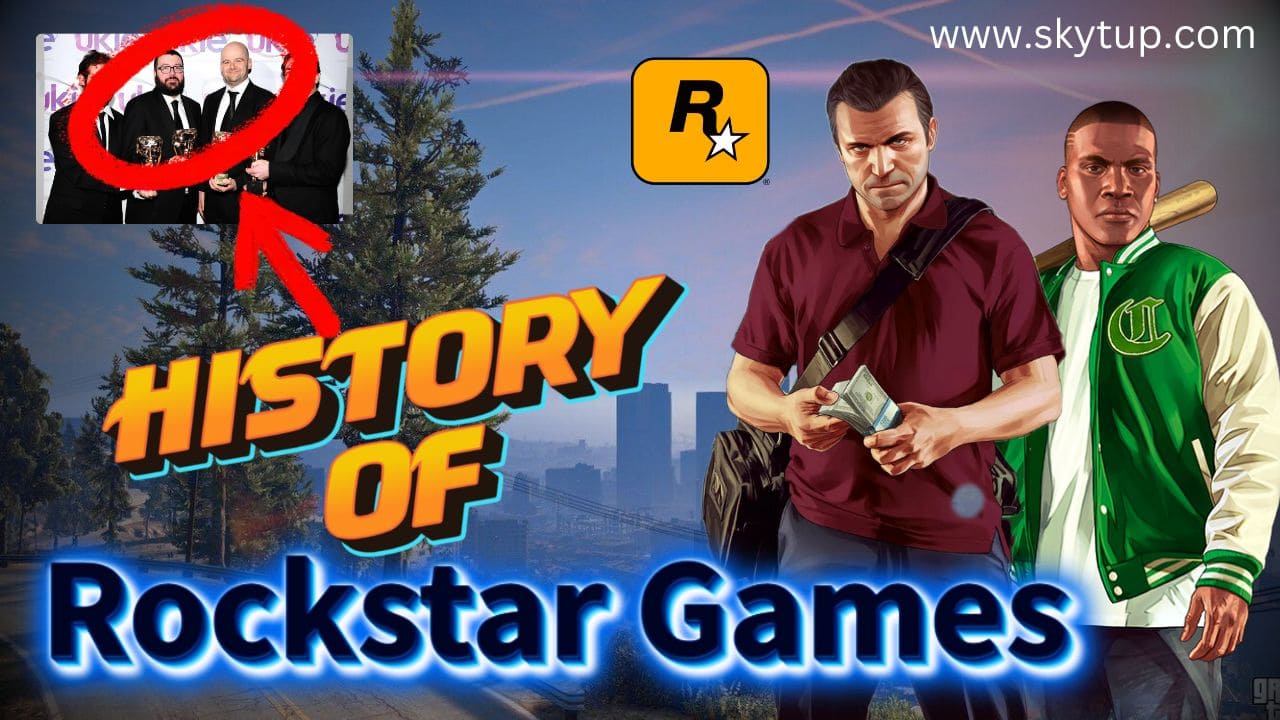
History of rockstar games in order | GTA V, GTA San Andrews, GTA Vice City, GTA IV
Rockstar Games, Inc. is an American video game publisher based in New York City. The company was established in December 1998 as a subsidiary of Take-Two Interactive, using the assets Take-Two had pre
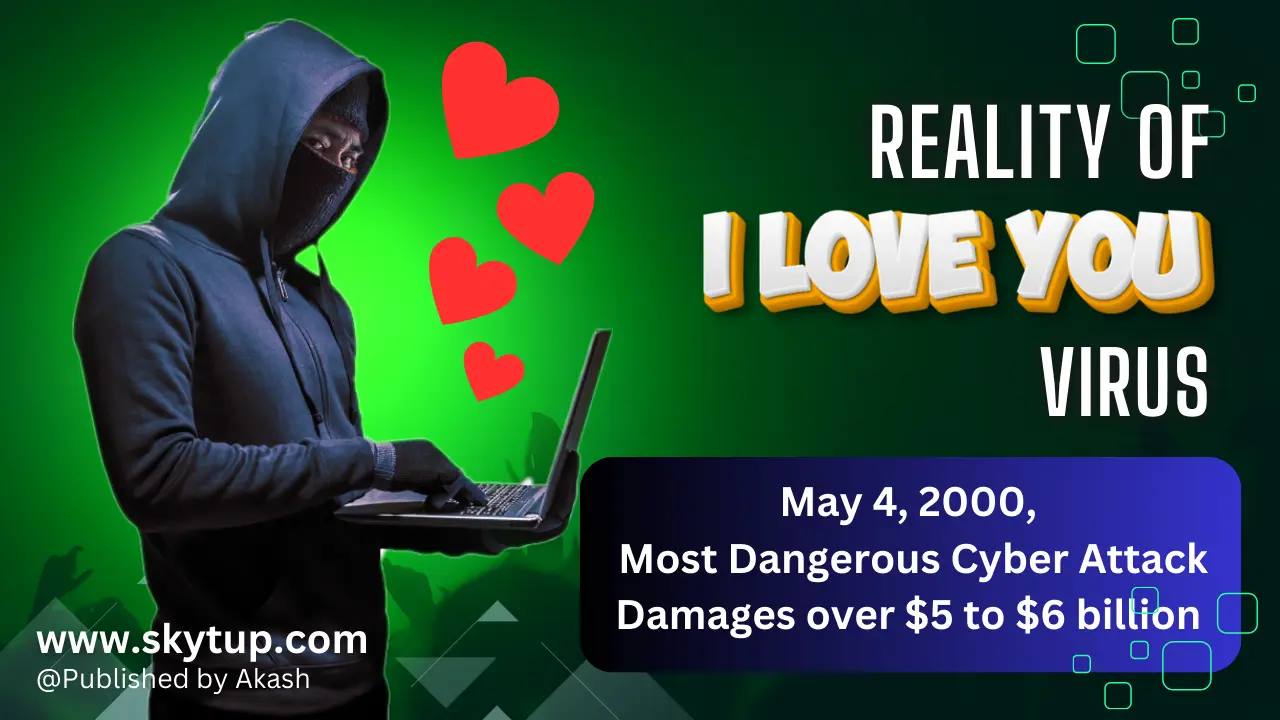
The I LOVE YOU Virus : A Historic Cyber Threat and How to Protect Yourself Today | Source Code
Discover the story behind the infamous ILOVEYOU virus, which caused billions in damages by exploiting email systems. Learn how this attack changed cybersecurity forever and get essential tips to prote
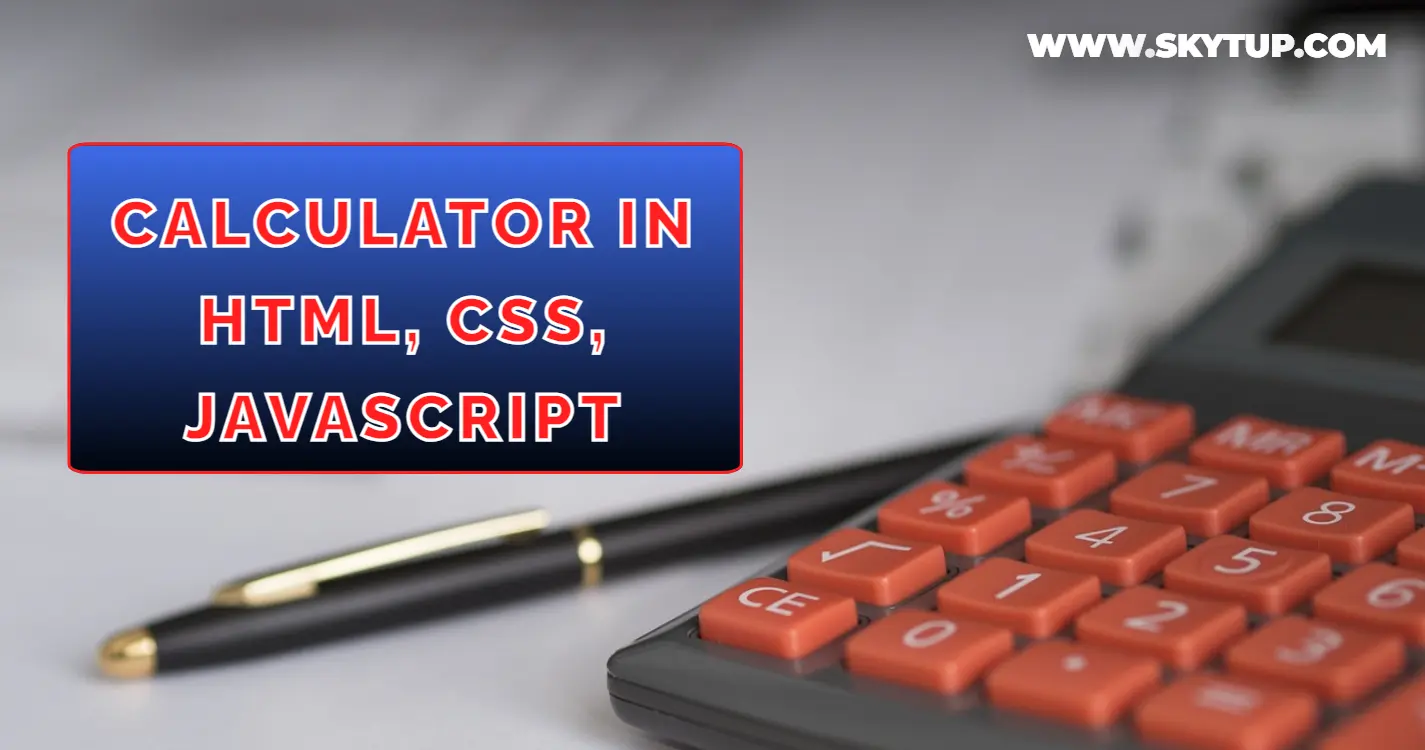
Lets Make a Simple Calculator using Html, Css, JavaScript | Source Code β
Creating an HTML Calculator using HTML, CSS, and JS Build a basic calculator using HTML, CSS, and JavaScript. Create the calculator&amp;#039;s layout with HTML, style it with CSS, and add func
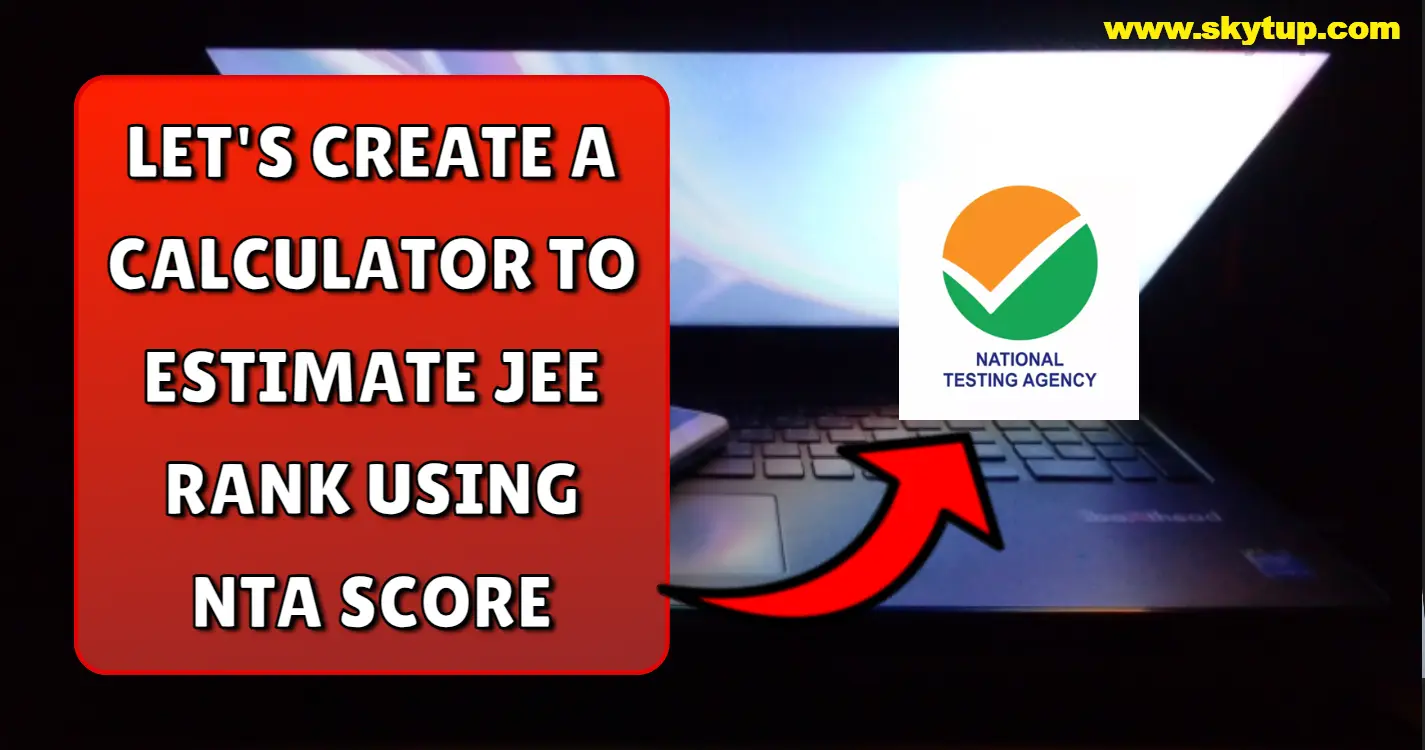
Lets Make a JEE Rank Calculator: Estimate Your 2024 JEE Mains Rank with HTML, CSS, and JavaScript
Discover how to build a JEE Rank Calculator using HTML, CSS, and JavaScript. This step-by-step guide helps aspiring engineers estimate their JEE Mains 2024 rank based on NTA scores. Learn to create an
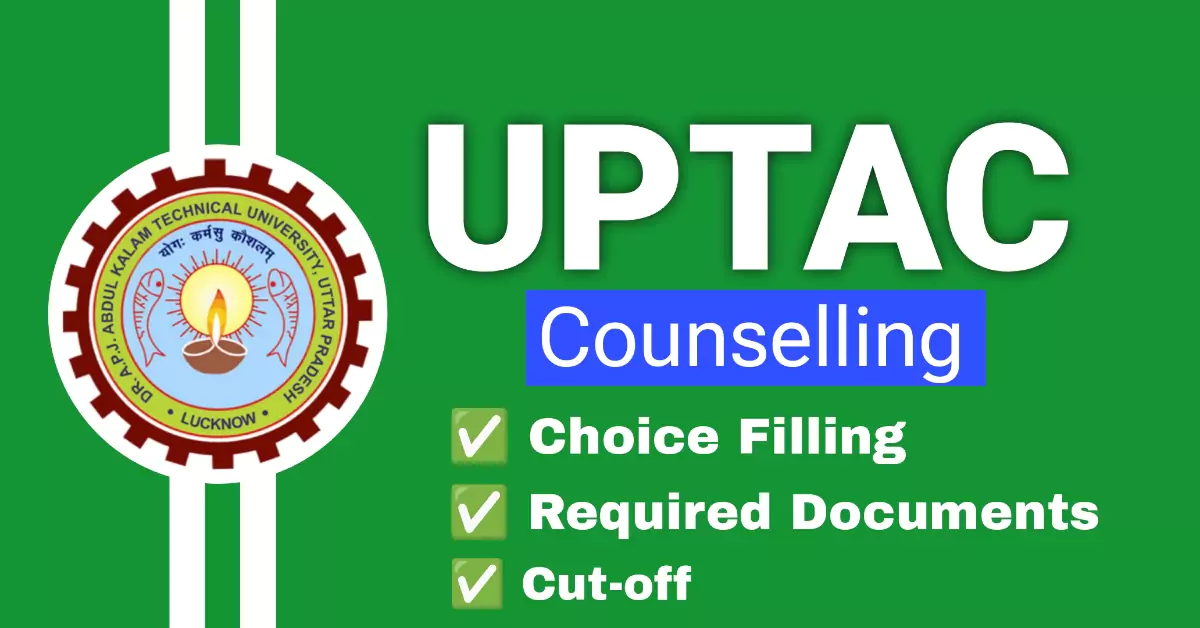
AKTU Counselling Choice FIlling BTECH 2024 | UPTAC Counselling Jee Mains | Engineering College 2024
This comprehensive guide explores the top 20 engineering colleges under AKTU for Computer Science and Engineering (CSE) and Information Technology (IT). Detailed information includes courses, fees, op
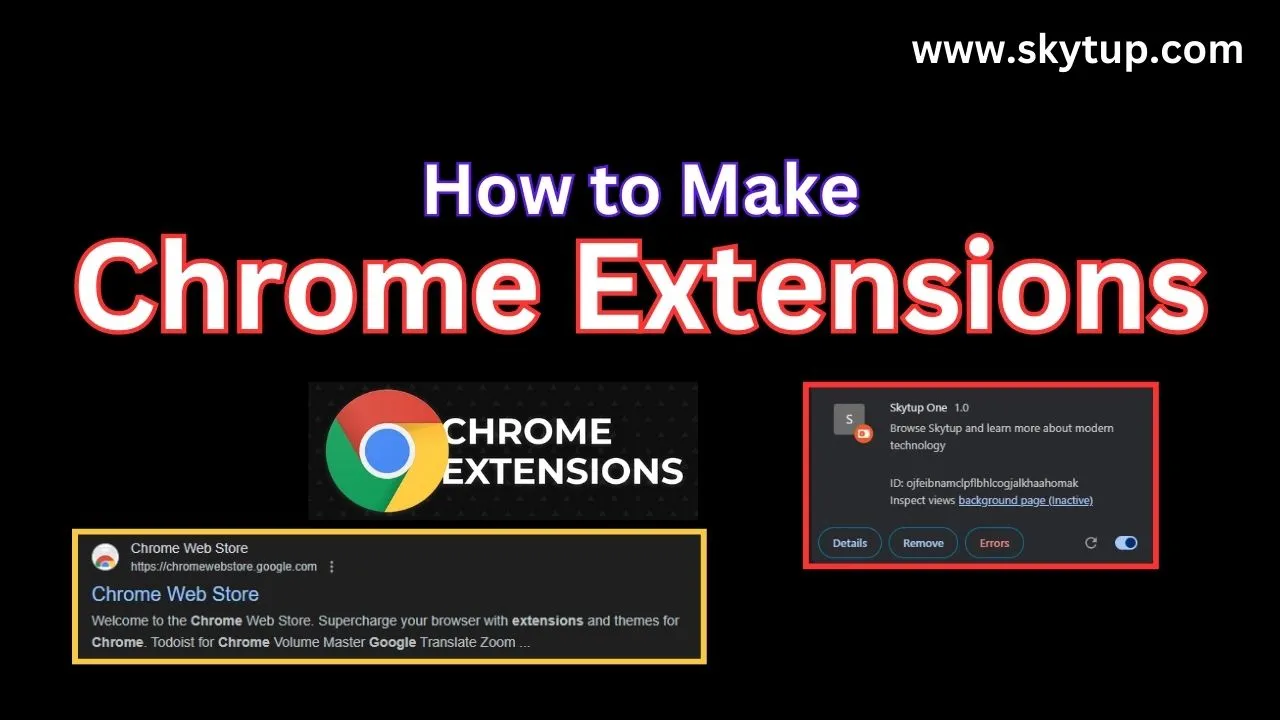
How to create google Chrome Extension in javascript for personal or Professional use
Creating Chrome extensions is a powerful way to enhance the web browsing experience and solve real-world problems. From simple UI modifications to complex integrations with web services, the possibili
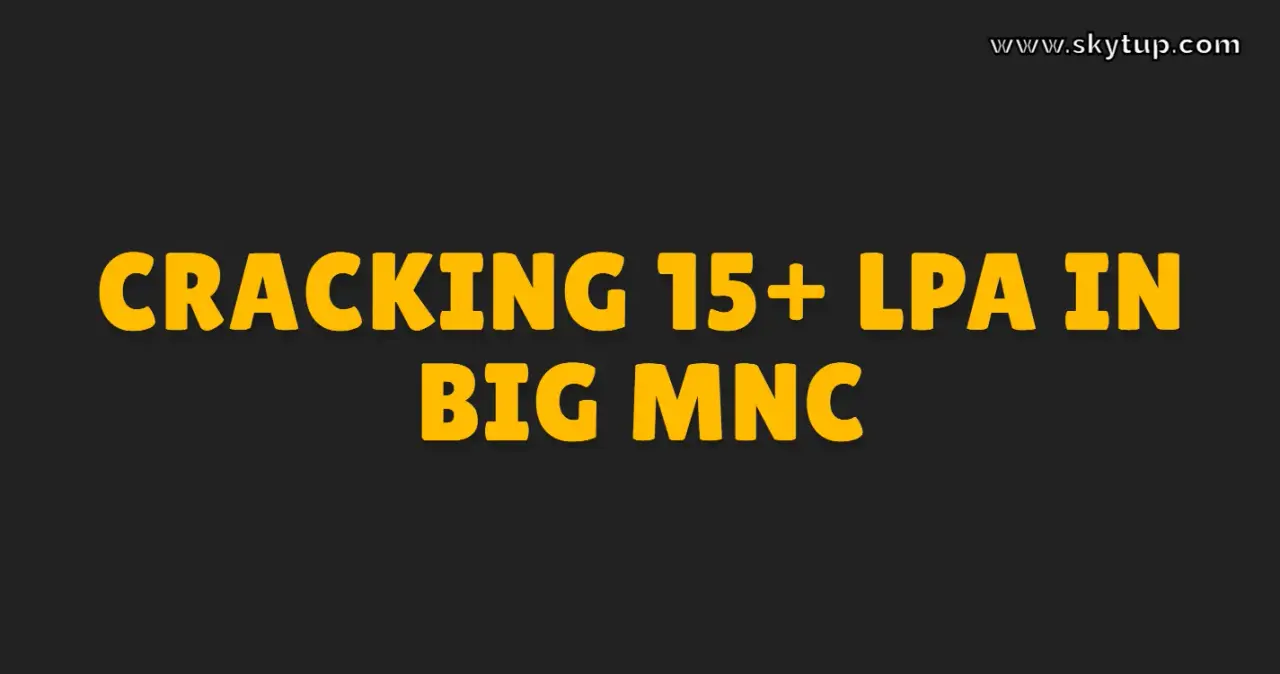
Cracking the 15+ LPA Code: Ultimate Guide to Securing Top MNC Placements
Unlock the secrets to landing high-paying jobs at leading MNCs with our comprehensive guide. From mastering data structures and algorithms to acing system design interviews, we cover it all. Learn to
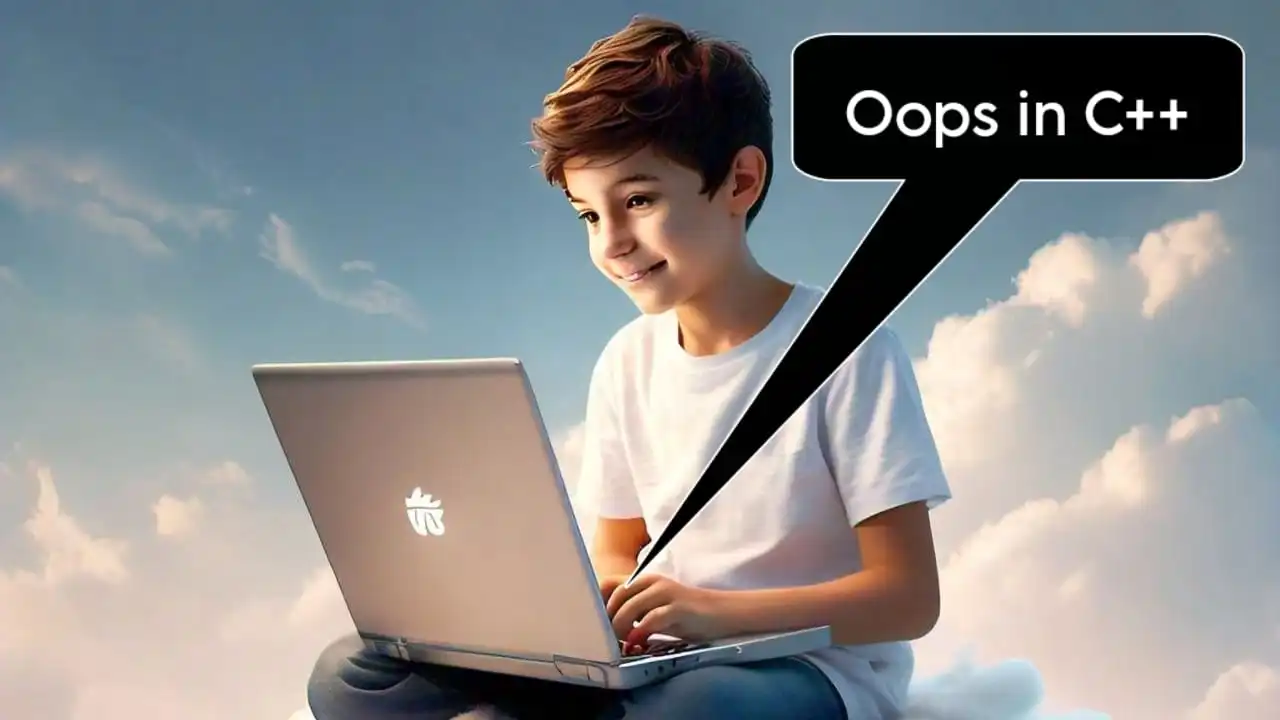
OOPs (Object Oriented Programming System) | Concepts & Interview Question with Examples
The major purpose of C++ programming is to introduce the concept of object orientation to the C programming language. Object Oriented Programming is a paradigm that provides many concepts such as inhe
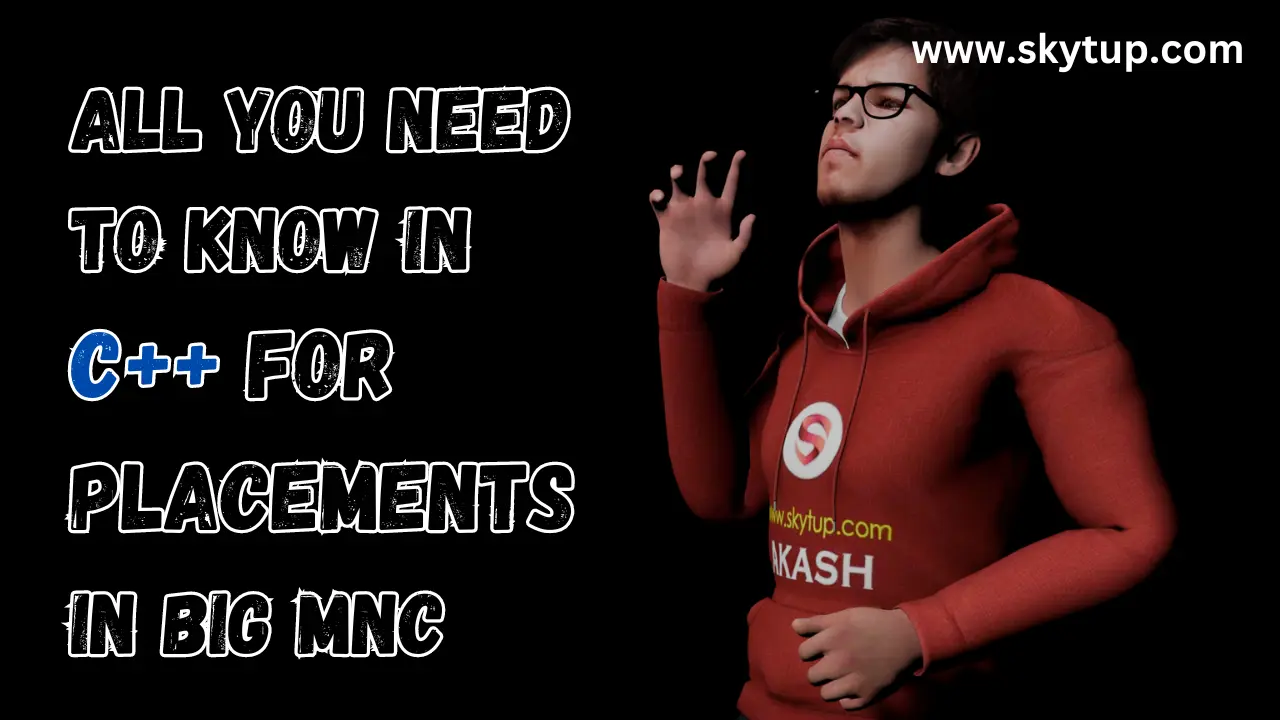
All you need to know in C++ for placement in big MNC | Requirements and eligibility criteria
Boost your chances of landing a job at top MNCs with expertise in C++. Learn the required skills, data structures, algorithms, and eligibility criteria to excel in placement tests. Stay ahead with our
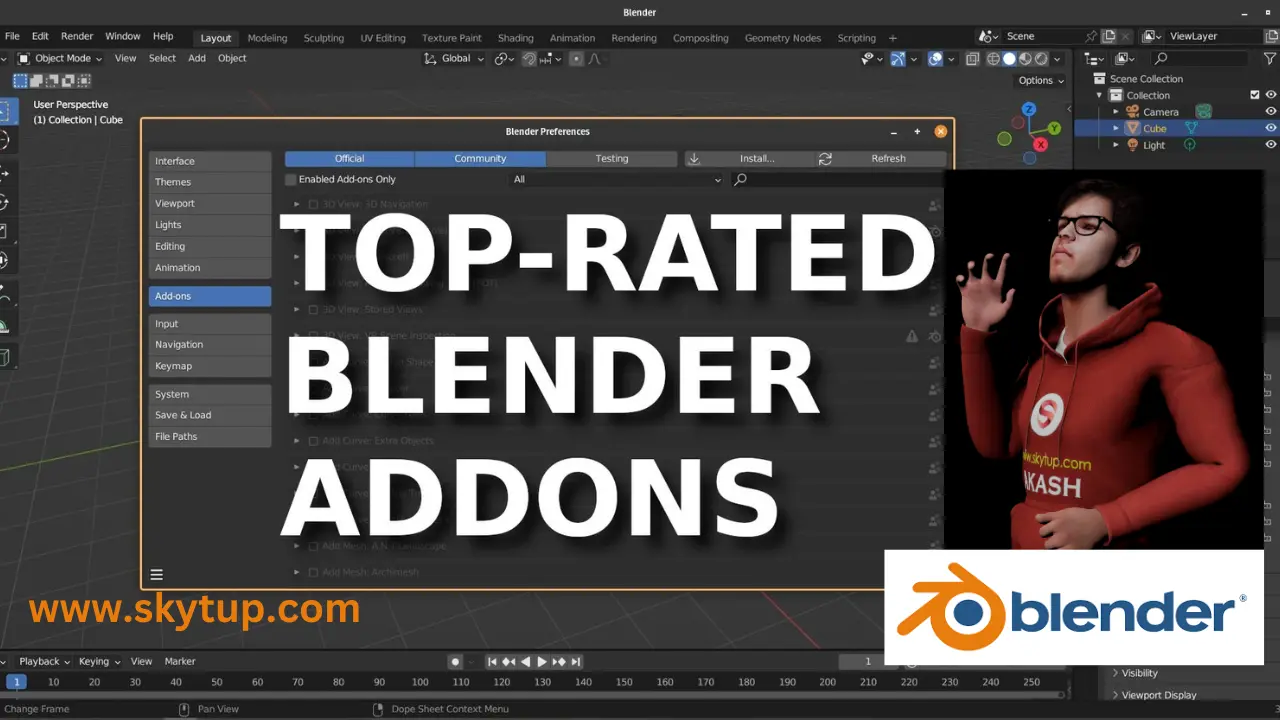
Top 15 Blender Add-ons for Becoming a Pro in the 3D World π₯
"Take your 3D skills to the next level with these top 10 Blender add-ons. From modeling and texturing to animation and rendering, discover the best tools to streamline your workflow and create s
Performance Comparison of Programming Languages: Counting from 1 to 1 Billion
This article compares the performance of various programming languages, including C, C++, Java, Python, Go, C#, and JavaScript, by measuring the time each takes to count from 1 to 1 billion. Detailed
LeetCode Problem π₯ 1945 - Sum of Digits of String After Convert
This type of problem is common in competitive programming and technical interviews, as it tests your understanding of basic string manipulation, numeric transformations, and iterative processes. In th

Building a Distance-Measuring LED Indicator Using Arduino and Ultrasonic Sensor π₯
Learn to create a distance-measuring LED indicator using an Arduino and an HC-SR04 ultrasonic sensor. This project guides you through setting up the sensor to control LEDs based on object proximity, o
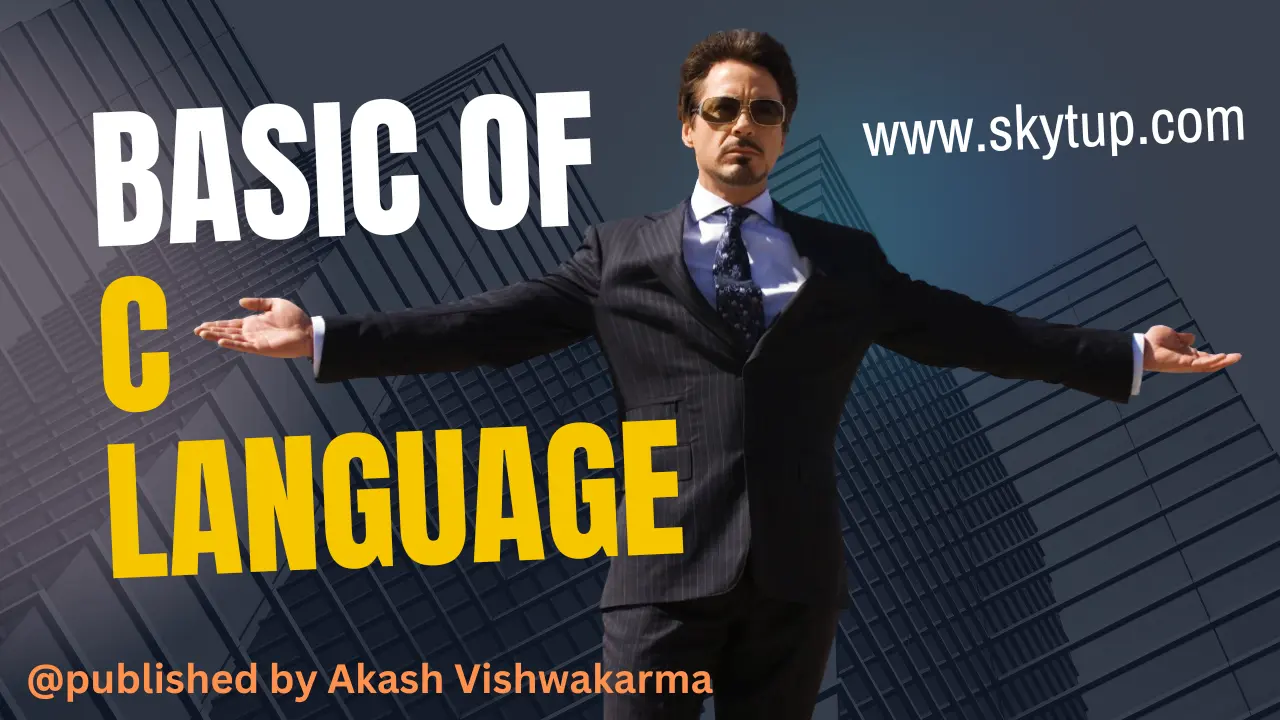
Basics of C Language for beginners | Syntax and Common Interview questionπ₯
C is a procedural programming language with a static system that has the functionality of structured programming, recursion, and lexical variable scoping. C was created with constructs that transfer w
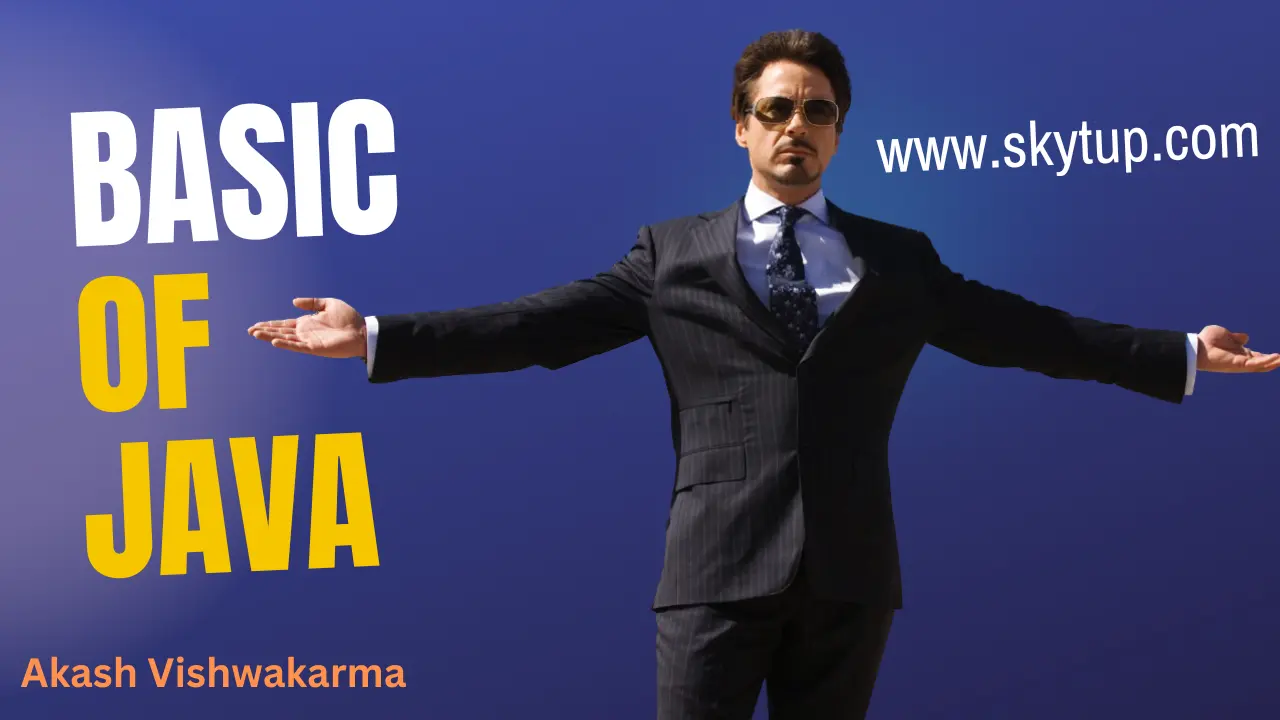
Basics of Java Programming and common Interview Questions π₯
Java Platform is a collection of programs. It helps to develop and run a program written in the Java programming language. Java Platform includes an execution engine, a compiler and set of libraries.
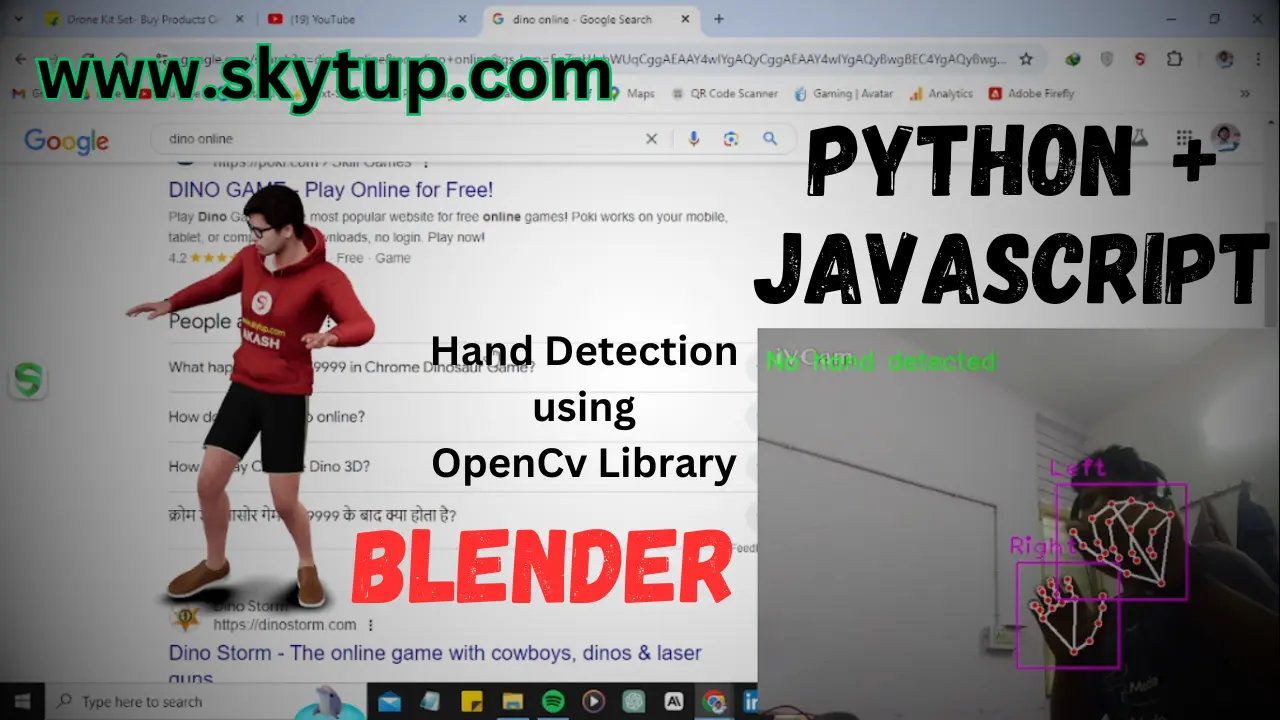
Hand Gesture Control Using OpenCV and Cvzone | Python Project by Akash Vishwakarma
Hey I am Akash Vishwakarma in this article I am gonna show you hand detection system using python with opencv library. This is an open source library which you can use to detect multiple hand gestures
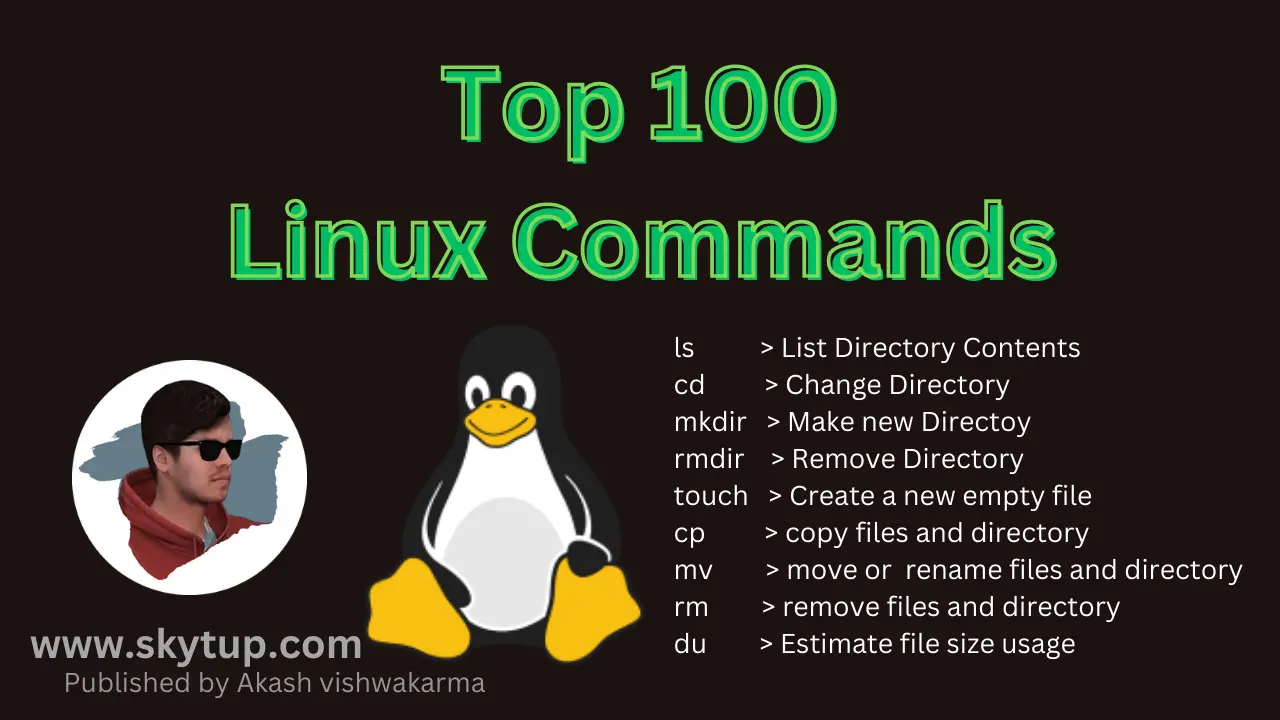
Top 100 Linux Commands you Must know in 2024 | Akash
Hi Everyone, My name is Akash in this article I you will learn top 100 linux commands. By mastering these essential Linux commands, you'll become more efficient and confident in your ability to w
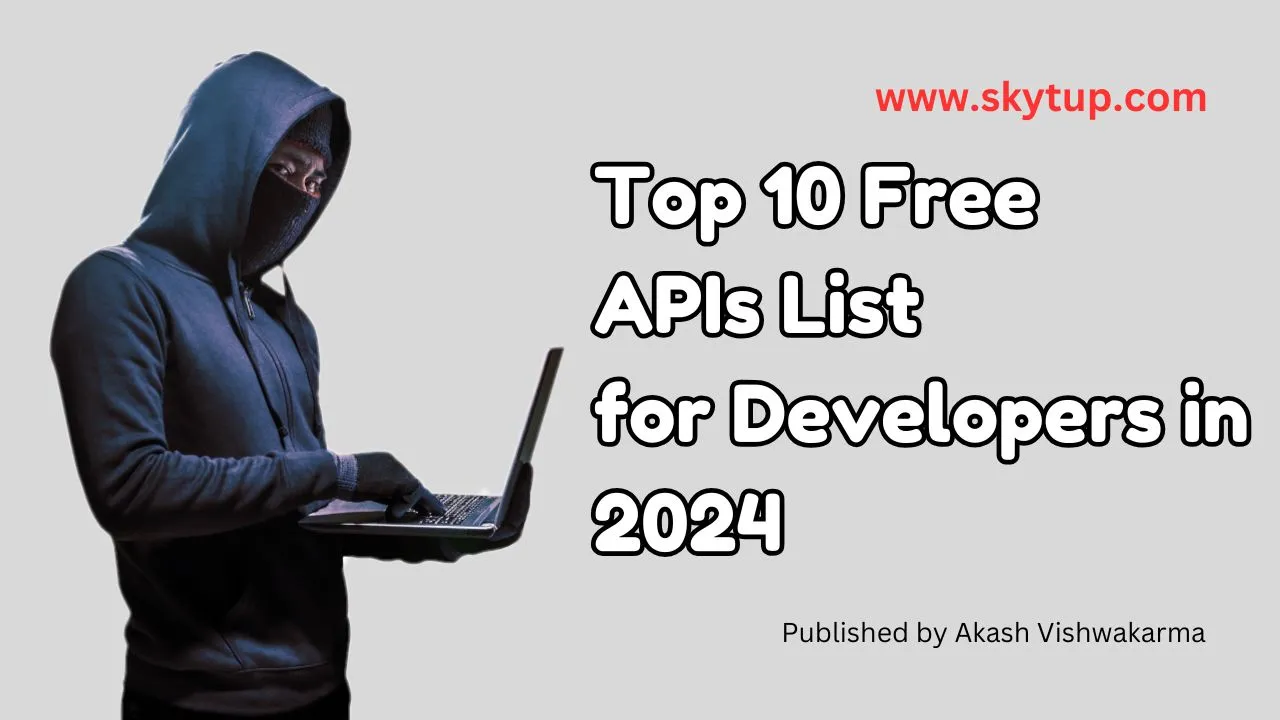
Top 10 Free APIs with Documentation for Developers in 2024 π₯
Hi Everyone this is Akash Vishwakarma. In this Article we have Top 10 Api List by using these Api Endpoints You can make Amazing Projects and showcase them on your portfolio. You can show these to rec
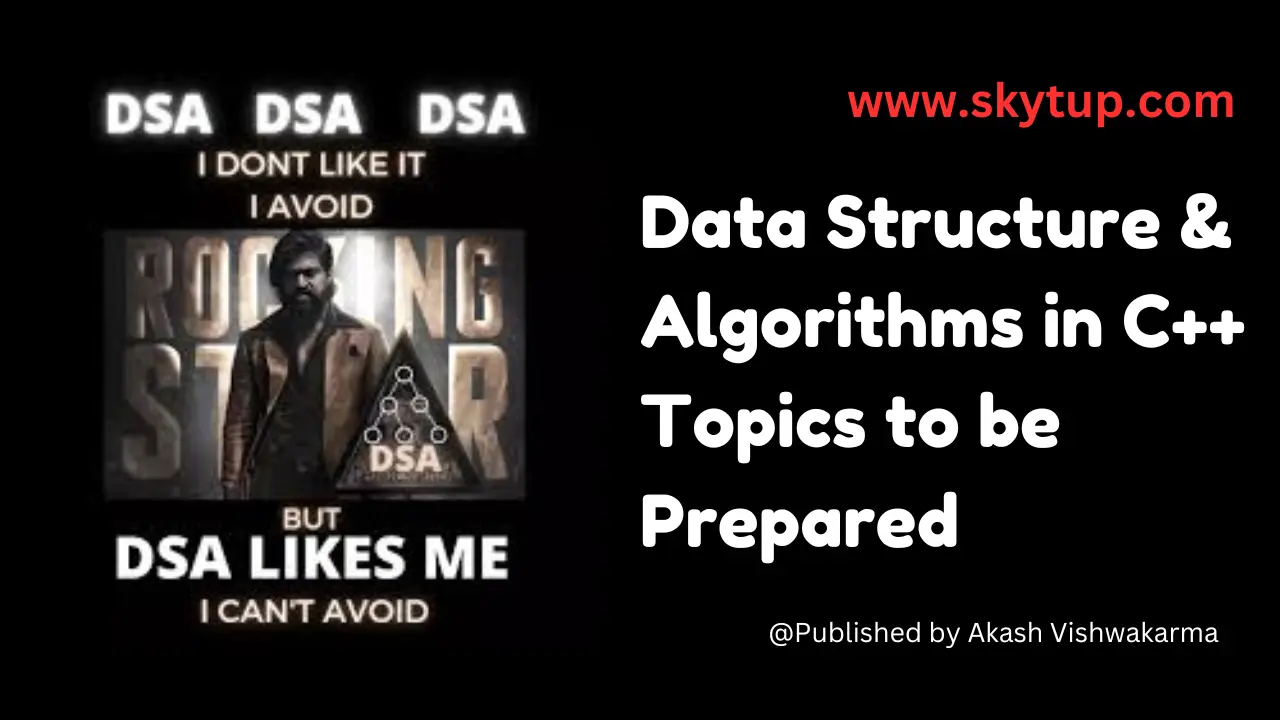
Data Structures and Algorithms in C++ | Topics to be prepared for Interviews
Hi everyone, I'm Akash Vishwakarma. As someone who passionate about programming, I've often found myself wondering what it takes to crack those tough technical interviews at top tech
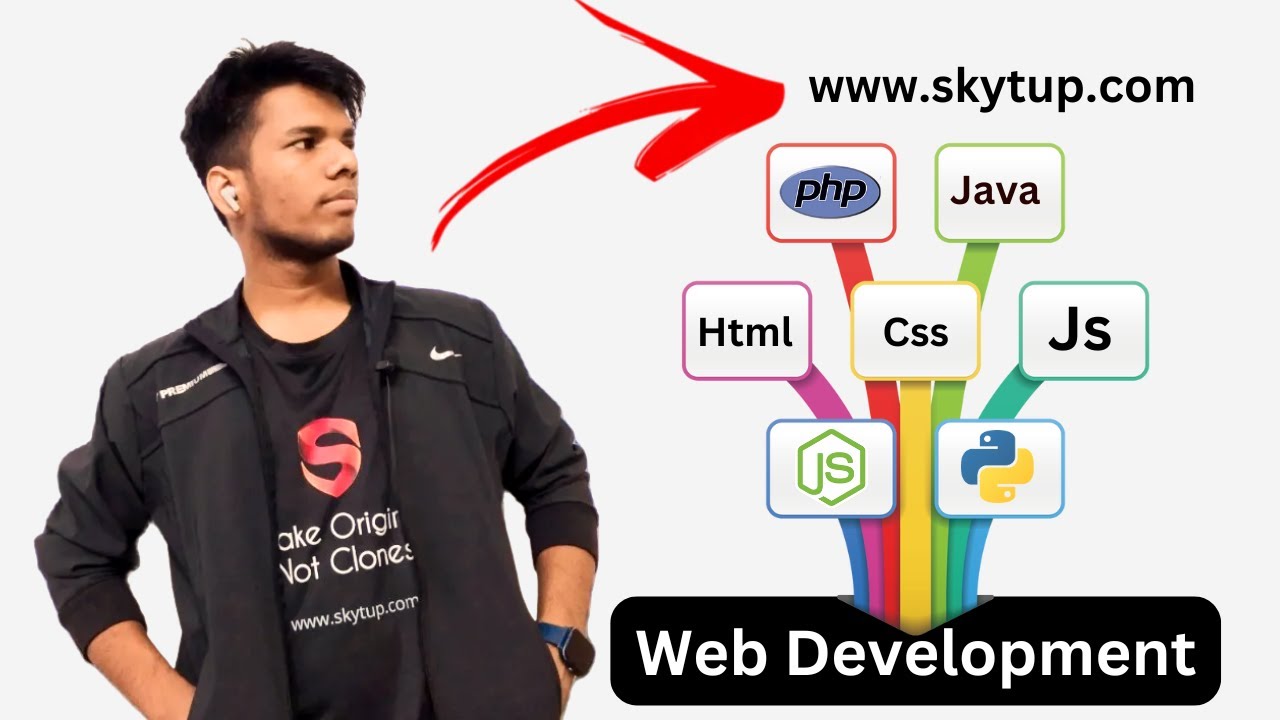
Become a Full Stack Web Developer | A Quick Roadmap for Beginners in 2025 | Developer Akash
Hi Everyone,its me Akash, In this Article I&amp;#039;ve Shared a Roadmap to become a Full Stack Web Developer. Follow guildines given in this article and you will must know something new and i

Advanced Guide to C++ STL for Competitive Programming
The C++ STL is built on three fundamental components: containers, algorithms, and iterators. Understanding how these components interact and their underlying implementations is crucial for optimal usa
#1 - Basic Fundamentals - DSA Series With C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is 1st Article of DSA Series on Skytup. In this Article We will learn about C++ Fundamentals. This article is written with the help of Ai Tools and some of my own exp
#2 - Arrays and Strings - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #2 of Our DSA Series on Skytup. In this Article We will learn about C++ Fundamentals. This article is written with the help of Ai Tools and some of my o
#3 - Linked Lists - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #3 of Our DSA Series on Skytup. In this Article We will learn about Linked Lists. This article is written with the help of Ai Tools and some of my own e
#4 - Stacks and Queues - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #4 of Our DSA Series on Skytup. In this Article We will learn about C++ Stack and Queues. This article is written with the help of Ai Tools and some of
#5 - Trees - Data Structures and Algorithms using C++ | Akash Vishwakarma
Hi its me Akash Vishwakarma, This is Article No #5 of Our DSA Series on Skytup. In this Article We will learn about C++ Trees. This article is written with the help of Ai Tools and some of my own expe